Matplotlib는 파이썬에서 사용할 수 있는 데이터 시각화 라이브러리 중 하나입니다. 초보자이든 전문가이든 관계없이 사전 지식이 없더라도 파이썬에서 Matplotlib를 사용하는 방법에 대해 소개합니다. 한 번에 다 읽으려다간 숨 넘어갈 수 있습니다(스압주의!).
권현욱(엑셀러) | 아이엑셀러 닷컴 대표 · Microsoft MVP · 엑셀 솔루션 프로바이더 · 작가
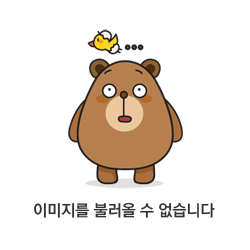
※ 이 글은 아래 기사 내용을 토대로 작성되었습니다만, 필자의 개인 의견이나 추가 자료들이 다수 포함되어 있습니다.
- 원문: Master Matplotlib: A Step-by-Step Guide for Beginners to Experts
- URL: https://medium.com/@hi-sushanta/master-matplotlib-a-step-by-step-guide-for-beginners-to-experts-e76195edff1f
Matplotlib 불러오기
먼저, Matplotlib 라이브러리를 PC로 불러옵니다.
import matplotlib
import matplotlib.pyplot as plt
# Check version
print(matplotlib.__version__)
# Output > 3.7.1
첫 번째 plot을 만들어 보겠습니다.
plt.plot();
첫 번째 플롯을 만든 것을 축하합니다! 이제 이 빈 플롯에서 무슨 일이 일어나고 있는지 알아볼 수 있도록 몇 가지 세부 사항을 살펴보겠습니다.
아래 이미지를 살펴보십시오. Matplotlib 플롯의 구조를 보여줍니다.
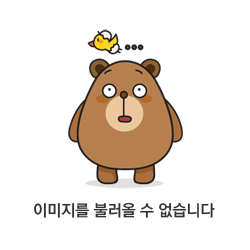
명심해야 할 두 가지 핵심 사항
그림: 그림은 그리는 모든 것을 포함하는 기본 창 또는 페이지입니다. 아래 예시를 확인하면 더 잘 이해할 수 있습니다.
plt.plot()
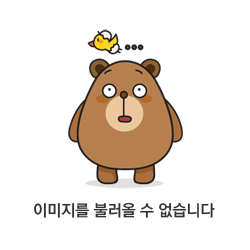
축: 이 그림 내에서 축을 통합합니다. 축은 플롯할 데이터 요소를 지정하는 영역을 정의합니다. 시각적 가이드는 아래 이미지를 참조하세요.
number_one = [1, 2, 3, 4, 5, 6, 7, 8, 9]
number_two = [10, 20, 30, 40, 50, 60, 70, 80, 90]
# Plotting the data
plt.plot(number_one, number_two);
# Adding labels to the axes
plt.xlabel('X-axis Label');
plt.ylabel('Y-axis Label');
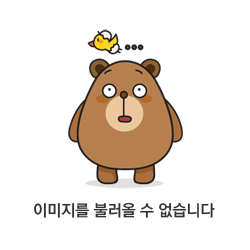
기억할 사항: 위의 예에서 모든 플롯에는 두 개의 축 X와 Y가 포함됩니다.
- X축은 'number_one'을 나타냅니다.
- Y축은 'number_two'를 나타냅니다.
# This step is common to every Matplotlib workflow, so be sure to keep it in mind.
# 1. Import matplotlib libray in your notebook
import matplotlib.pyplot as plt
# 2. Generate or use existing data for your visualization.
first_number = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12]
secound_number = [11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22]
# 3. Configure the plot settings.
fig, ax = plt.subplots(figsize=(6,6))
# 4. Plot data
ax.plot(first_number, secound_number)
# 5. Customize plot
ax.set(title="This is my numbers plot", xlabel="x-axis", ylabel="y-axis")
# 6. Save your plot & show
fig.savefig("/content/sample_data")python
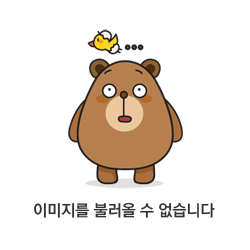
'This is my Numbers Plot'이라는 제목의 플롯을 성공적으로 만들었습니다. 이제 이 플롯을 구성하는 데 사용한 코드 뒤에 숨은 의미를 알아볼 시간입니다. 각 코드 조각을 단계별로 분석해 보겠습니다.
import matplotlib.pyplot as plt
Matplotlib 라이브러리로 작업할 때 가장 먼저 해야 할 일은 노트북으로 라이브러리를 가져오는 것입니다. 명령은 다음과 같습니다.
import matplotlib
이 코드를 이해해 볼까요.
.pyplot as plt
pyplot은 Matplotlib 라이브러리입니다. 그리고 Matplotlib은 Python의 2D 데이터 시각화 라이브러리입니다. 이 라이브러리는 john D. Hunter가 만들었습니다. Matplotlib은 Matlab과 유사한 인터페이스를 사용할 수 있도록 설계되었습니다. 이 라이브러리의 가장 큰 장점 중 하나는 무료 오픈 소스이기 때문에 누구나 이 라이브러리를 사용하고 구현할 수 있다는 것입니다.
as plt # plt is shortfrom is pyplot
"""
It's not mandatory to use plt. You can define a shorter name
that you find easy to remember.
"""
다음 코드를 이해해 봅시다.
"""
This is my data, I create myself. So I can build a plot.
- first_number — X axis
- secound_number — Y axis
"""
first_number = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12]
secound_number = [11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22]
# 3. Setup plot
fig, ax = plt.subplots(figsize=(6,6))
subplot( )은 하나의 그림 안에 여러 개의 플롯을 만들 수 있는 Matplotlib의 함수입니다. 또한 figsize( )는 인수를 전달하여 그림(또는 플롯)의 크기를 지정할 수 있는 Matplotlib의 메서드입니다. 아래 코드 예시를 참조하면 더 잘 이해할 수 있습니다.
import numpy as np
# Sample data for stock prices
days = np.arange(1, 11)
stock_price_A = np.random.rand(10) * 10 + 50 # Generating random prices for stock A
stock_price_B = np.random.rand(10) * 8 + 45 # Generating random prices for stock B
# Creating subplots
fig, axs = plt.subplots(2, 1, figsize=(10, 8))
# Plotting stock prices on the first subplot
axs[0].plot(days, stock_price_A, label='Stock A', marker='o', color='blue')
axs[0].set_title('Stock Price of A')
axs[0].set_xlabel('Days')
axs[0].set_ylabel('Price')
axs[0].legend()
# Plotting stock prices on the second subplot
axs[1].plot(days, stock_price_B, label='Stock B', marker='s', color='green')
axs[1].set_title('Stock Price of B')
axs[1].set_xlabel('Days')
axs[1].set_ylabel('Price')
axs[1].legend()
# Adjusting layout for better spacing
plt.tight_layout()
# Display the plot
plt.show()
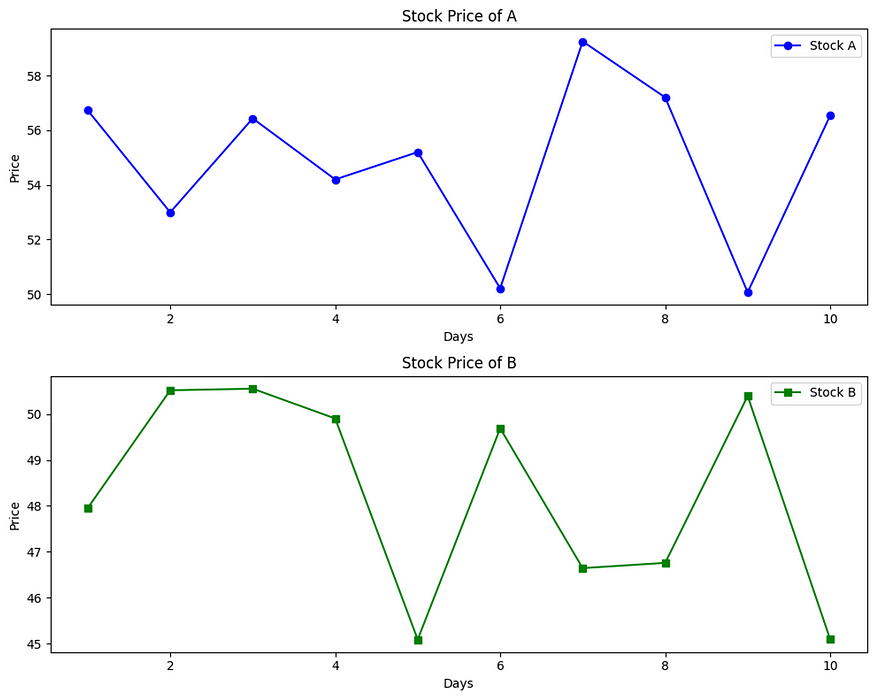
Matplotlib은 NumPy 배열을 기반으로 구축되었습니다. 따라서 이 섹션에서는 NumPy 배열을 사용할 때 가장 일반적인 유형의 플롯을 배웁니다. 이 섹션에서는 이 주제를 다룹니다.
- Line
- Scatter
- Bar
- 히스토그램
- 파이
- 서브플롯 ( )
이제 먼저 NumPy 라이브러리를 가져옵니다.
import numpy as np
꺾은선형 차트를 배우기 전에 Matplotlib 마커(marker)의 개념을 파악하는 것이 중요합니다. 이러한 이해는 Matplotlib 라이브러리 내의 모든 플롯에 대한 이해도를 높여줄 것입니다.
Matplotlib Marker
point_y = np.array([2, 8, 4, 12])
plt.plot(point_y, marker = 'o')
plt.show()
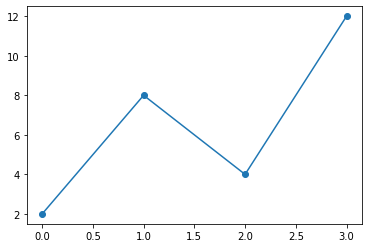
마커는 플롯에서 시각적 지표 역할을 하므로 특정 관심 지점을 강조 표시할 수 있습니다. 다음 예에서는 포인트 2, 4, 8, 12를 표시했습니다. 보다 명확한 시각화를 보려면 위 이미지를 참조하세요. 플롯에서 포인트를 표시하려면 마커 키워드를 활용할 수 있습니다.
point_y = np.array([2, 8, 4, 12])
plt.plot(point_y, marker = '*');
몇 가지 마커 참조 목록은 [여기]에서 확인할 수 있습니다. 이제 플롯을 사용자 지정할 차례입니다.
point_y = np.array([2, 8, 4, 10])
plt.plot(point_y, 'o:r') # o is a mareker and r is a red
plt.show()
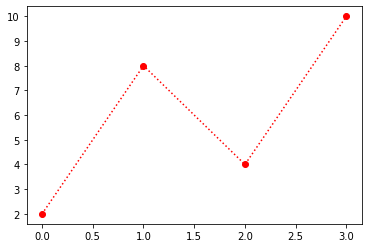
다른 것을 시도해 봅니다. 이번에는 녹색입니다.
point_y = np.array([2, 8, 4, 10])
plt.plot(point_y, 'o:g')
plt.show()
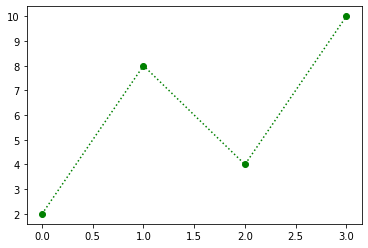
다음은 마커의 색상 참조 사항 중 일부입니다.
- ‘r’ — Red🔴
- ‘g’ — Green 🟢
- ‘b’ — Blue🔵
- ‘y’ — Yellow 🟡
- ‘k’ — Black ⚫
Matplotlib에서 마커 크기 변경
point_y = np.array([3, 8, 1, 10])
plt.plot(point_y, marker = 'o', ms = 21) # ms is short for — Markersize
plt.show()
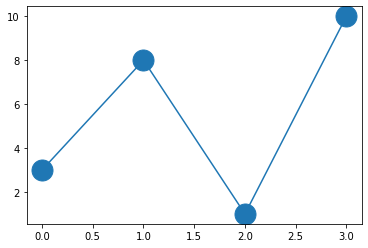
위의 예시에서 녹색 플롯의 마커 크기를 조정합니다.
point_y = np.array([2, 8, 4, 10])
plt.plot(point_y, 'o:g', ms = 21)
plt.show()
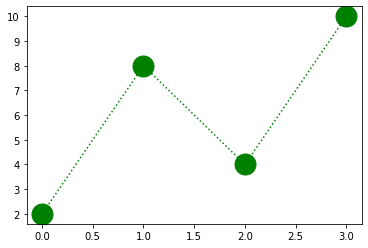
마커 가장자리의 색상을 지정합니다.
point_y = np.array([2, 6, 1, 10])
plt.plot(point_y, marker = 'o', ms = 20, mec = 'r')
plt.show()
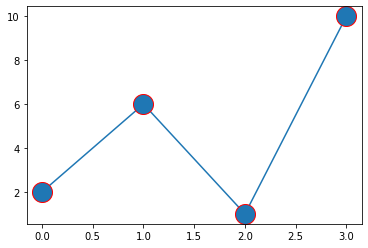
위의 코드를 이해해 봅시다.
plt.plot(point_y, marker = 'o', ms = 20, mec = 'r')
- marker: 플롯에서 포인트를 표시하는 데 사용되는 원, 사각형 또는 삼각형과 같은 기호입니다.
- ms: 마커 심볼의 크기를 결정하는 마커 크기입니다. 이 예에서는 마커 크기를 20으로 사용했습니다.
- mec(마커 가장자리 색상): 마커의 윤곽선 색상입니다. 약어 'mec'는 '마커 가장자리 색상'을 의미합니다. 위의 예에서는 마커 가장자리 색상으로 빨간색을 사용했습니다.
Marker 페이스 색상 변경
이 섹션에서는 마커 가장자리 색상을 변경하는 방법, 즉 마커의 내부 색상을 변경하는 방법에 대해 알아봅니다.
point_y = np.array([3, 8, 1, 10])
plt.plot(point_y, marker = 'o', ms = 20, mfc = 'r')
# mfc — Is short form marker face color.
plt.show()
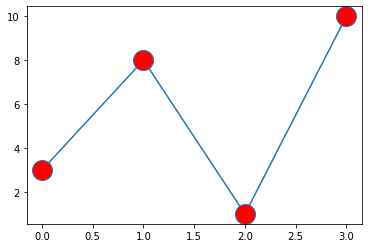
한 가지 예를 더 봅니다.
point_y = np.array([2, 8, 1, 10])
plt.plot(point_y, marker = 'o', ms = 21, mfc = 'y')
plt.show()
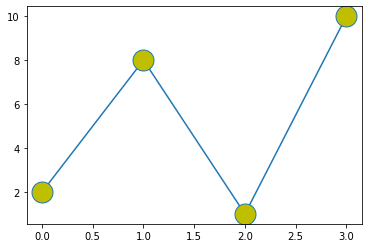
이제 마커 가장자리(mec)와 마커 면 색상(mfc)을 모두 적용해 보겠습니다.
point_y = np.array([3, 8, 4, 9,6])
plt.plot(point_y, marker = 'o', ms = 16, mec = 'r', mfc = 'r')
plt.show()
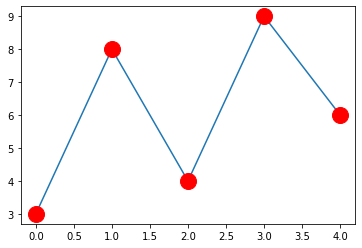
이제 마커의 개념을 이해하셨으니 간단한 연습을 통해 지식을 실습해 보겠습니다. 과제는 다음과 같습니다:
- 원하는 마커 세 개를 선택합니다.
- 마커 크기를 30으로 조정합니다.
- 마커 면의 색을 노란색으로 설정합니다.
Matplotlib를 사용해 꺾은선형 차트 만드는 방법
이 섹션에서는 Matplotlib로 인상적인 꺾은선형 차트를 만드는 방법을 단계별로 배웁니다.
point_y = np.array([2, 8, 4, 10])
plt.plot(point_y, linestyle = 'dotted')
plt.show()
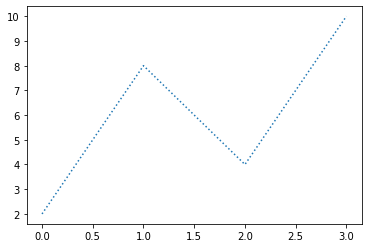
코드를 이해해 봅니다.
plt.plot(point_y, linestyle = 'dotted')
Matplotlib의 linestyle을 사용하면 꺾은선형 차트의 표시 방식을 조정할 수 있습니다. 'linestyle'이라는 전체 단어 또는 약어 'ls'를 사용할 수 있습니다. 실선, 점선, 점선 등 다양한 스타일이 있습니다. 예를 들어 점선이 필요한 경우 선 스타일을 '점선'으로 설정하거나 'ls='dotted''을 사용합니다. 선의 모양을 사용자 정의하는 것이 전부입니다.
point_y = np.array([2, 8, 4, 10])
plt.plot(point_y, ls = 'dotted') # this time i use ls
plt.show()
몇 가지 꺾은선형 차트 스타일을 살펴봅니다.
point_y = np.array([2, 8, 4, 10])
plt.plot(point_y, ls = 'dashed') # i use dashed style
plt.show()
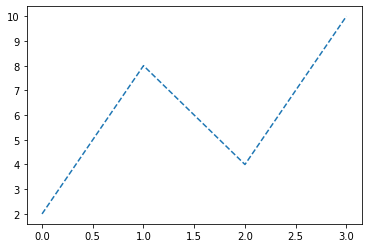
point_y = np.array([2, 8, 4, 10])
plt.plot(point_y, ls = 'dashdot') # i use dashdot style
plt.show()
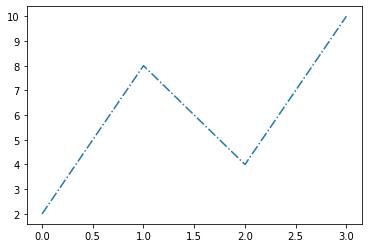
다양한 선 스타일에 대해 더 자세히 알아보고 싶다면 [여기]에서 더 많은 인사이트와 세부 정보를 확인하세요.
Matplotlib에서 선 색상을 변경하는 방법
아래 코드 예제를 살펴보고 Matplotlib에서 선 색상을 사용자 지정하는 방법을 알아보세요.
point_y = np.array([2, 8, 4, 10])
plt.plot(point_y, color = 'g')
plt.show()
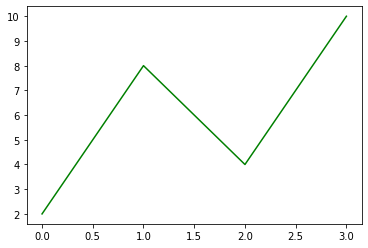
코드를 살펴봅니다.
plt.plot(point_y, color = 'g')
color는 Matlpotlib에서 선 색을 그릴 때 사용하는 키워드입니다. color는 짧은 형식의 c입니다. color 또는 c를 모두 사용할 수 있습니다. 아래 예시를 참고하세요.
point_y = np.array([2, 8, 4, 10])
plt.plot(point_y, c = 'g') # this time i use c keyword
plt.show()
또는 16진수 색상 값을 사용할 수도 있습니다.
point_y = np.array([2, 8, 4, 10])
plt.plot(point_y, c = '#FFD700')
plt.show()
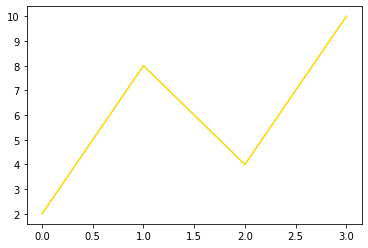
Matplotlib에서 선 너비 변경
Matplotlib으로 선 너비를 손쉽게 조정하여 플롯의 시각적 매력을 향상시키는 방법을 알아봅니다.
point_y = np.array([2, 8, 4, 10])
plt.plot(point_y, linewidth = '16.3')
plt.show()
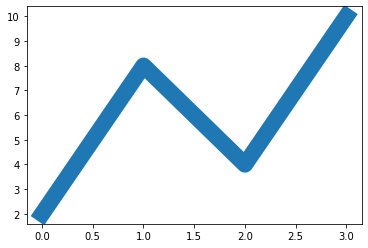
plt.plot(point_y, linewidth = '16.3')
linewidth는 선의 너비를 조정할 수 있는 Matplotlib의 키워드입니다. 선폭의 짧은 형태는 lw입니다. 선폭의 작동 원리를 이해하기 위해 다른 예를 살펴보겠습니다.
point_y = np.array([2, 8, 4, 10])
plt.plot(point_y, c = '#00ff83', lw = '9') # this time i use lw
plt.show()
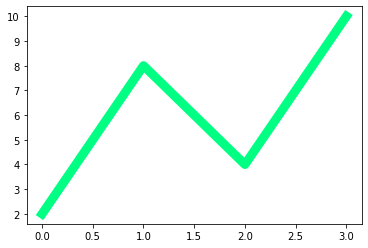
plt.plot() 함수를 사용하여 두 개의 선을 단일 플롯으로 결합해 보겠습니다.
first_line = np.array([2, 8, 4, 10])
secound_line = np.array([6, 2, 12, 14])
plt.plot(first_line, c = 'r')
plt.plot(secound_line, c = "g")
plt.show()
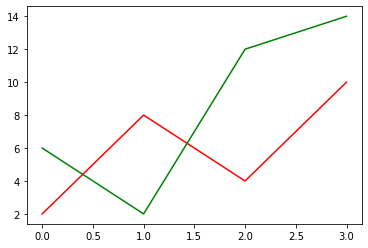
이 시점에서 Matplotlib에서 플롯을 만드는 기본 사항을 마스터하셨습니다. 다음 단계는 사용자 지정 기술을 익히는 것입니다. 플로팅을 그림의 한 형태라고 생각하고 시각적으로 매력적인 데이터 표현을 만드는 것을 목표로 하세요. 플롯을 보기 좋게 만드는 것은 예술 작품을 만드는 것과 같으며, 이를 공유하면 사람들이 여러분의 작품을 좋아할 것입니다.
Matplotlib의 플롯에 레이블과 제목을 추가하는 방법
x = np.array ([1, 2, 3, 4, 5, 6, 7, 8])
y = np.array ([9, 10, 11, 12, 13, 14, 15, 16])
plt.plot(x, y)
plt.title("This is my - Numbers plot");
plt.xlabel ("This is my X axis - first number ");
plt.ylabel ("This is my Y axis - and secound number");
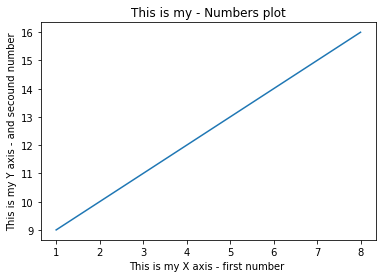
위의 코드를 분석해 보겠습니다. 필자는 PyPlot 메서드를 사용하여 데이터를 플롯합니다. PyPlot 을 사용할 때 xlabel( ) 및 ylabel( ) 함수를 사용하여 플롯의 x축과 y축에 각각 레이블을 지정할 수 있습니다. 또한 title( ) 함수는 플롯의 제목을 설정하는 데 사용됩니다.
- x축의 경우: xlabel( )
- y축의 경우: ylabel()
이러한 함수를 자유롭게 탐색하여 플롯 라벨링 기술을 향상시키세요.
Myplotlib 플롯에서 글꼴 스타일과 크기 변경
x = np.array ([1, 2, 3, 4, 5, 6, 7, 8])
y = np.array ([9, 10, 11, 12, 13, 14, 15, 16])
font1 = {'family':'serif','color':'red','size':18}
font2 = {'family':'serif','color':'green','size':14}
font3 = {'family':'serif','color':'blue','size':14}
# In this line of code, I create font name, color, and font size
plt.title("This is my - Numbers plot", fontdict = font1);
plt.xlabel ("This is my X axis - first number ", fontdict = font2);
plt.ylabel ("This is my Y axis - and secound number", fontdict = font3);
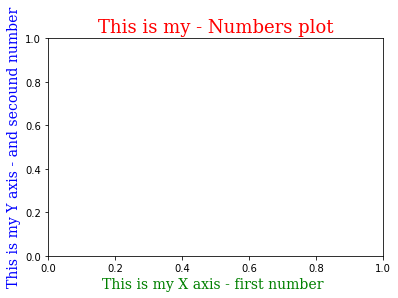
fontdict()는 PyPlot의 편리한 함수입니다. 이제 제목의 위치를 조정하는 방법을 살펴보겠습니다.
x = np.array ([1, 2, 3, 4, 5, 6, 7, 8])
y = np.array ([9, 10, 11, 12, 13, 14, 15, 16])
font1 = {'family':'serif','color':'red','size':16}
font2 = {'family':'serif','color':'green','size':14}
font3 = {'family':'serif','color':'blue','size':14}
plt.title("This is my - Numbers plot", fontdict = font1, loc = 'left');
plt.xlabel ("This is my X axis - first number ", fontdict = font2);
plt.ylabel ("This is my Y axis - and secound number", fontdict = font3);
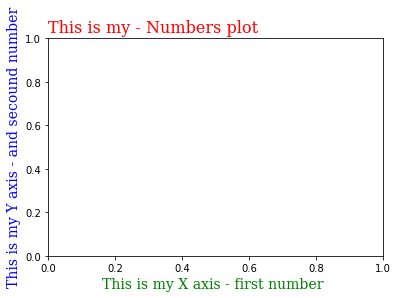
loc 매개변수를 사용하여 제목의 위치를 변경할 수 있습니다. 왼쪽, 오른쪽, 가운데 값을 사용할 수 있습니다. 기본값은 가운데입니다. 다른 예시를 참조하면 더 잘 이해할 수 있습니다.
#define x and y
x = [1, 2, 3, 4, 5, 6]
y = [7, 8, 9, 10, 11, 12]
#create plot of x and y
plt.plot(x, y)
#add title
plt.title('1-12 Numbers Plot', loc='left');
plt.title('I Understand', loc ='right');
Matplotlib 그림에 격자선 추가하기
x = [1, 2, 3, 4, 5, 6]
y = [7, 8, 9, 10, 11, 12]
plt.title ('This is my numbers line chart ');
plt.xlabel ('X axis ');
plt.ylabel ('Y axis ');
plt.plot(x, y);
plt.grid()
plt.show()
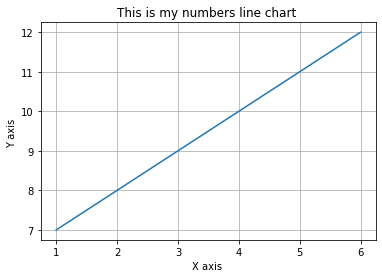
Pyplot에서는 grid( ) 함수를 사용하여 그리드를 꺾은선형 차트에 결합하기만 하면 됩니다. 기본적으로 X 및 Y 그리드 선이 모두 표시되지만, 이 설정을 유연하게 사용자 지정할 수 있습니다. 간단한 데모를 보려면 아래 코드 예시를 확인하세요.
x = [1, 2, 3, 4, 5, 6]
y = [7, 8, 9, 10, 11, 12]
plt.title ('This is my numbers line chart ');
plt.xlabel ('X axis ');
plt.ylabel ('Y axis ');
plt.plot(x, y);
plt.grid(axis = 'y') # This line show only Y grid
plt.show()
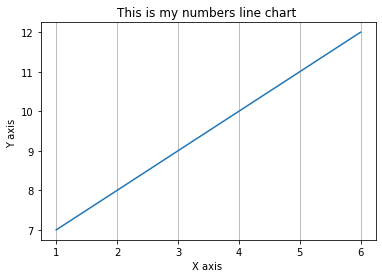
x = [1, 2, 3, 4, 5, 6]
y = [7, 8, 9, 10, 11, 12]
plt.title ('This is my numbers line chart ');
plt.xlabel ('X axis ');
plt.ylabel ('Y axis ');
plt.plot(x, y);
plt.grid(axis = 'y') # This line show only Y grid
plt.show()
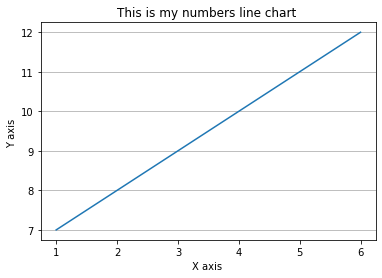
이제 그리드 스타일을 변경할 차례입니다. 아래 예시를 참조하세요.
x = [1, 2, 3, 4, 5, 6]
y = [7, 8, 9, 10, 11, 12]
plt.title ('This is my numbers line chart ');
plt.xlabel ('X axis ');
plt.ylabel ('Y axis ');
plt.plot(x, y);
plt.grid(color = 'red', linestyle = '--', linewidth = 0.5)
# Note — grid(color = 'color', linestyle = 'linestyle', linewidth = number).
plt.show()
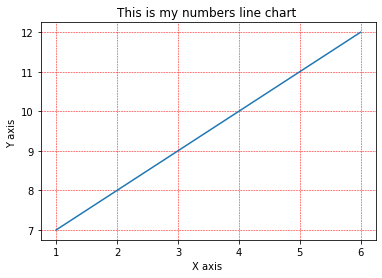
Matplotlib의 Subplot
이 섹션에서는 Matplotlib에서 서브플롯을 만드는 방법에 대해 자세히 알아보겠습니다. 코드를 살펴보기 전에 먼저 서브플롯이 정확히 무엇인지 이해해 보겠습니다.
Matplotlib에서 서브플롯이란 무엇인가요?
Matplotlib의 서브플롯은 하나의 그림 안에 여러 개의 플롯을 그릴 수 있는 기능입니다. 더 잘 이해하려면 아래 코드 예제를 살펴보세요.
"""
yfinance library is a Python library that provides access to financial
data from Yahoo Finance.It can be used to download historical and
current stock prices, options data, and other financial information.
"""
import yfinance as yf
# Fetch stock data for three companies
stock_data_company1 = yf.download('AAPL', start='2022-01-01', end='2023-01-01')
stock_data_company2 = yf.download('MSFT', start='2022-01-01', end='2023-01-01')
stock_data_company3 = yf.download('TSLA', start='2022-01-01', end='2023-01-01')
# Create subplots
fig, axs = plt.subplots(3, 1, figsize=(10, 12), sharex=True)
# Plot stock prices for Company 1 (AAPL) in black
axs[0].plot(stock_data_company1['Close'], label='AAPL', color='black')
axs[0].set_title('Stock Price - AAPL')
axs[0].legend()
# Plot stock prices for Company 2 (MSFT) in red
axs[1].plot(stock_data_company2['Close'], label='MSFT', color='red')
axs[1].set_title('Stock Price - MSFT')
axs[1].legend()
# Plot stock prices for Company 3 (TSLA) in blue
axs[2].plot(stock_data_company3['Close'], label='TSLA', color='blue')
axs[2].set_title('Stock Price - TSLA')
axs[2].legend()
# Set common xlabel
plt.xlabel('Date')
# Adjust layout for better appearance
plt.tight_layout()
# Show the plots
plt.show()
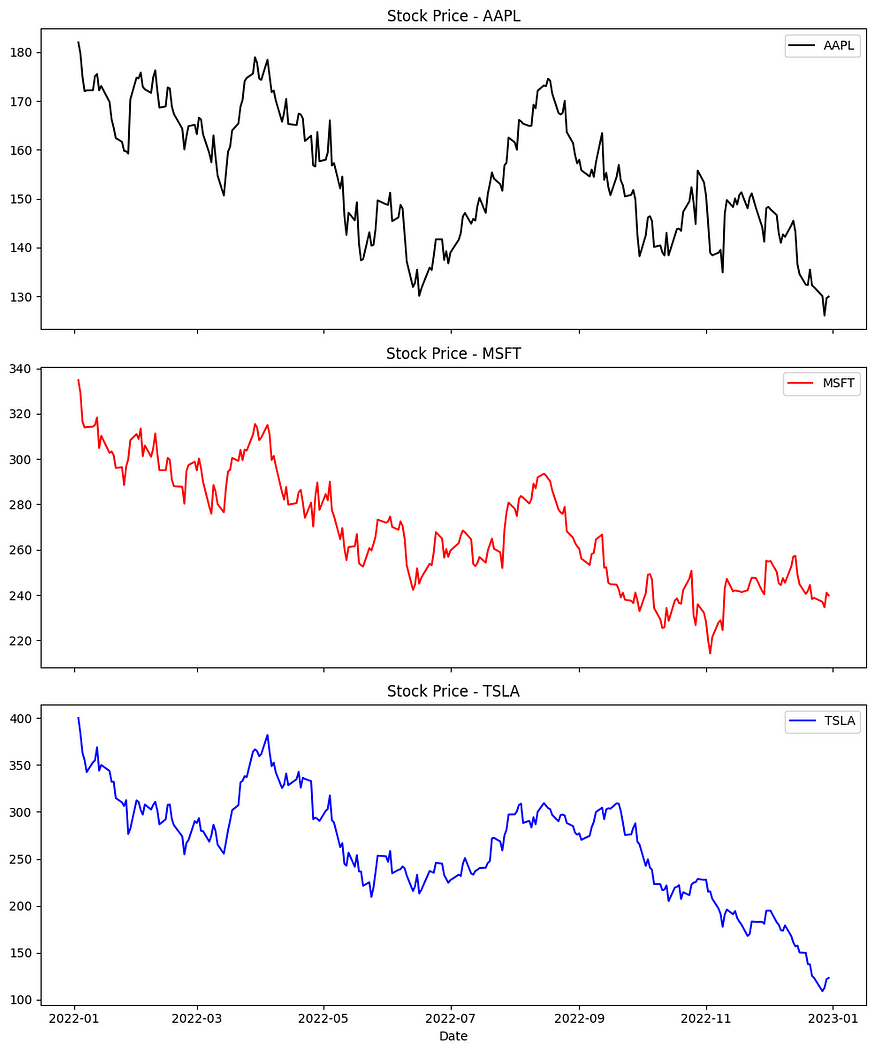
Subplot 함수 이해하기
subplot( ) 함수는 세 개의 인수를 받으며, 각 인수는 플롯을 정렬하는 데 중요한 역할을 합니다. 첫 번째 인수는 행의 수를 결정하고 두 번째 인수는 열의 수를 지정합니다. 세 번째 인수는 흔히 플롯 인덱스라고 하며 그리드 내에 표시할 플롯을 지정합니다. 동일한 코드를 살펴보겠습니다.
import yfinance as yf
import matplotlib.pyplot as plt
# Define the ticker symbols for Tesla and Apple
tesla_ticker = "TSLA"
apple_ticker = "AAPL"
# Retrieve historical stock data for Tesla and Apple
tesla_data = yf.download(tesla_ticker, period="5y")
apple_data = yf.download(apple_ticker, period="5y")
# Extract the closing prices from the historical data
tesla_prices = tesla_data["Close"]
apple_prices = apple_data["Close"]
# Create a new figure with two subplots
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(12, 6))
# Plot Tesla's stock prices in red color on the first subplot
ax1.plot(tesla_data.index, tesla_prices, color="red", label="Tesla")
ax1.set_title("Tesla Stock Price (5Y)")
ax1.set_xlabel("Date")
ax1.set_ylabel("Stock Price")
# Plot Apple's stock prices in black color on the second subplot
ax2.plot(apple_data.index, apple_prices, color="black", label="Apple")
ax2.set_title("Apple Stock Price (5Y)")
ax2.set_xlabel("Date")
ax2.set_ylabel("Stock Price")
# Adjust the layout of the subplots to avoid overlapping labels
fig.tight_layout()
# Add a legend to the overall figure
plt.legend(loc="upper left")
# Show the combined line chart
plt.show()
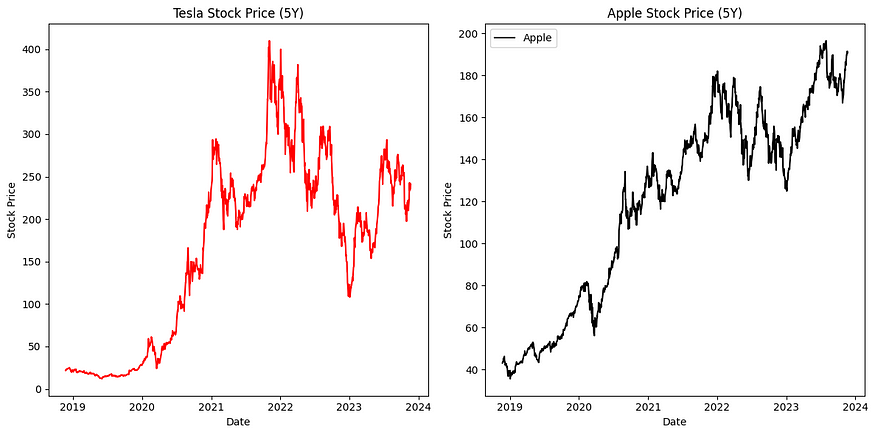
이제 plot에 제목을 추가합니다.
import yfinance as yf
import matplotlib.pyplot as plt
# Define the ticker symbols for Tesla and Apple
tesla_ticker = "TSLA"
apple_ticker = "AAPL"
# Retrieve historical stock data for Tesla and Apple
tesla_data = yf.download(tesla_ticker, period="5y")
apple_data = yf.download(apple_ticker, period="5y")
# Extract the closing prices from the historical data
tesla_prices = tesla_data["Close"]
apple_prices = apple_data["Close"]
# Create a new figure with two subplots
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(12, 6))
# Plot Tesla's stock prices in red color on the first subplot
ax1.plot(tesla_data.index, tesla_prices, color="red", label="Tesla")
# Set titles for the subplots (Tesla)
ax1.set_title("Tesla Stock Price (5Y)")
ax1.set_xlabel("Date")
ax1.set_ylabel("Stock Price")
# Plot Apple's stock prices in black color on the second subplot
ax2.plot(apple_data.index, apple_prices, color="black", label="Apple")
# Set titles for the subplots (Apple)
ax2.set_title("Apple Stock Price (5Y)")
ax2.set_xlabel("Date")
ax2.set_ylabel("Stock Price")
# Add an overall title to the figure
fig.suptitle("Comparison of Tesla and Apple Stock Prices (5Y)")
# Adjust the layout of the subplots to avoid overlapping labels
fig.tight_layout()
# Add a legend to the overall figure
plt.legend(loc="upper left")
# Show the combined line chart
plt.show()
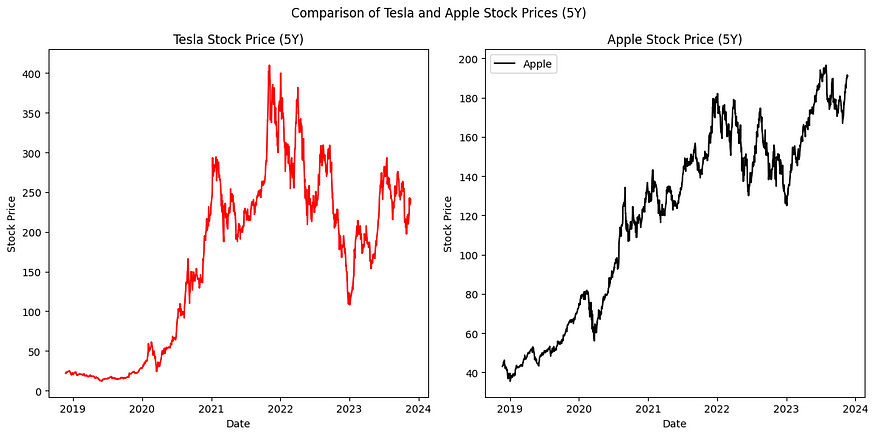
matplotlib의 서브플롯을 이해하셨다면 큰 문제가 되지 않을 것입니다. 이 블로그 게시물을 읽고 직접 코딩해 보세요. 조금만 연습하면 모든 것을 이해할 수 있을 것입니다.
산점도 Matplotlib
이제 Matplotlib에서 산점도를 그리는 방법을 배워보겠습니다. 하지만 그 전에 산점도(Scatter plot)가 무엇인지 이해해야 합니다. 참고로, 산점도를 분산형 차트라고도 합니다.
산점도는 점을 사용하여 데이터를 시각적으로 표현합니다. 산점도를 만들려면 길이가 같은 두 개의 배열이 필요합니다. 하나는 X축의 값을 위한 것이고 다른 하나는 Y축의 값을 위한 것입니다. 이해가 되셨나요? 그렇지 않더라도 걱정하지 마세요. 아래 예제 코드를 확인하면 더 명확하게 이해할 수 있습니다.
# Data
numbers = np.array([2, 3, 4, 5])
multiply_2 = np.array([4, 6, 8, 10])
# Create a scatter plot with red dots
plt.scatter(numbers, multiply_2, color='red', marker='o', label='Multiply by 2')
# Add grid
plt.grid(True, linestyle='--', alpha=0.7)
# Add labels and title
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Scatter Plot: Numbers vs. Multiply by 2')
# Show the plot
plt.show()
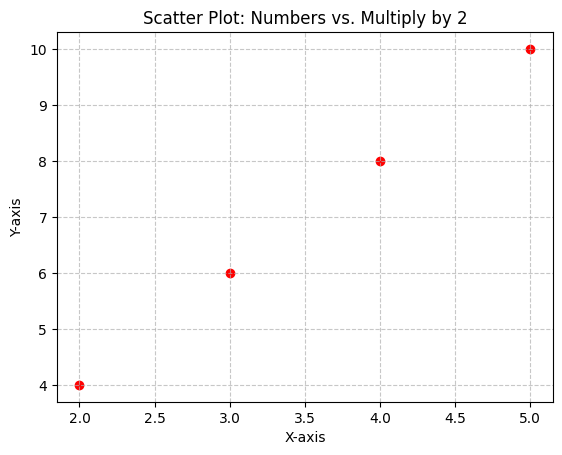
위의 코드를 분석해 보겠습니다. X축에는 일련의 숫자가 있습니다. 해당 Y축 값은 각 숫자에 2를 곱한 결과인 [4, 6, 8, 10]을 나타냅니다. 이제 한 가지 예를 더 보겠습니다.
# Data
years = np.array([2010, 2012, 2014, 2016, 2018, 2020])
bank_assets = np.array([500, 600, 750, 900, 1100, 1300])
# Create a scatter plot with blue dots and grid
plt.scatter(years, bank_assets, color='blue', marker='o', label='Banking Growth')
plt.grid(True, linestyle='--', alpha=0.7)
# Add labels and a title
plt.xlabel('Year')
plt.ylabel('Bank Assets (in Billion USD)')
plt.title('Banking Growth Over the Years')
# Show the plot
plt.show()
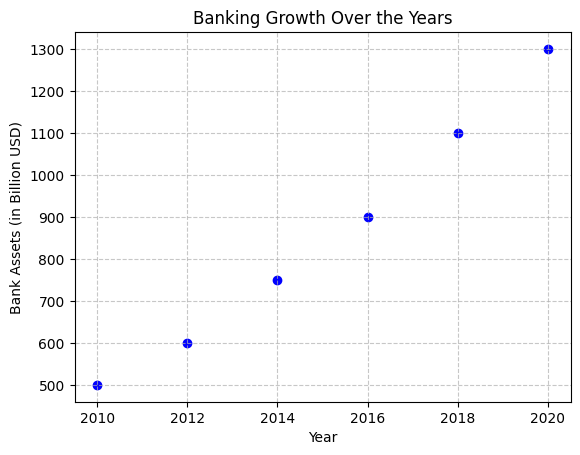
위의 분산형 차트에서는 수년에 걸친 은행의 성장을 시각화하고 있습니다. X축은 2010년부터 2020년까지의 연도를 나타내고, Y축은 해당 은행의 자산을 억 달러 단위로 나타냅니다. 플롯의 각 점은 은행의 재무 상태의 스냅샷을 나타내며, 지정된 기간 동안 은행의 성장 궤적을 관찰하고 분석할 수 있습니다. 점의 상승 추세는 플러스 성장을 의미하며, 수년 동안 은행의 자산이 증가했음을 보여줍니다.
비교를 위해 다른 차트를 만들어 보겠습니다. 다음은 컴퓨터 과학과 수학의 연도별 시험 합격 보고서를 비교하는 분산형 차트의 예입니다.
# Data (adjusted to showcase changes)
years = np.array([2015, 2016, 2017, 2018, 2019, 2020])
cs_pass_rate = np.array([75, 80, 85, 90, 92, 95])
math_pass_rate = np.array([80, 80, 85, 80, 90, 80]) # Math pass rate increases in 2017 and 2019
# Create a scatter plot with red dots for Computer Science and circular dots for Math
plt.scatter(years, cs_pass_rate, color='red', marker='o', label='Computer Science')
plt.scatter(years, math_pass_rate, color='black', marker='o', facecolors='none', edgecolors='black', label='Math')
# Add grid
plt.grid(True, linestyle='--', alpha=0.7)
# Add labels and a title
plt.xlabel('Year')
plt.ylabel('Passing Rate (%)')
plt.title('Yearly Exam Passing Report: Computer Science vs. Math')
# Add a legend
plt.legend()
# Show the plot
plt.show()
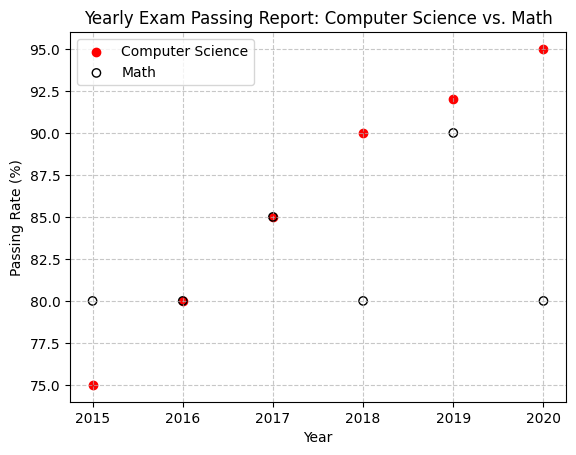
산점도의 각 점에 서로 다른 색상 지정
산점도의 모든 점 색상을 변경할 수 있습니다.
# This is a computer science exam passing data
years = np.array([2008, 2012, 2014, 2018, 2022])
pass_exam_computer_science = np.array([80, 77, 67, 56, 46])
# Remove yellow and add orange
colors = np.array(["red", "green", "black", "orange", "gray"])
plt.scatter(years, pass_exam_computer_science, c=colors, marker='o', s=50) # Specify marker style and size
plt.title("This is yearly report computer science exam")
plt.xlabel("Years")
plt.ylabel("Passing percentage")
plt.grid()
plt.show()
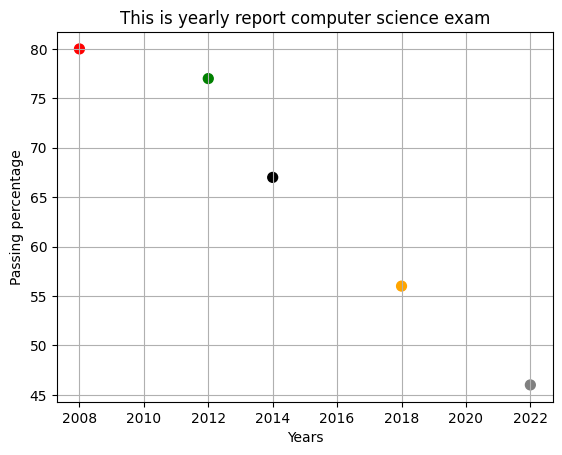
이제 광범위한 색상 목록을 제공하는 Matplotlib의 컬러맵 모듈의 환상적인 세계로 들어가 보겠습니다. 이 목록의 각 색상 값은 0에서 100까지 다양합니다. 개념을 더 잘 이해하기 위해 아래 예시를 살펴보겠습니다.

위에 보이는 이미지의 이름은 '비리디스'이며, 보라색 0에서 노란색 100까지의 색상 범위가 특징입니다. 🟣🟡 이제 궁금하실 겁니다.
Matplotlib 컬러맵을 적용하는 방법
아래 예시를 통해 더 명확하게 이해할 수 있습니다.
# This is a computer science exam passing data
years = np.array([2008, 2012, 2014, 2018, 2022])
pass_exam_computer_science = np.array([80, 77, 67, 56, 46])
colors = np.array ([10, 20, 30, 50, 60 ])
"""
To apply a specific colormap, you can use the cmap keyword.
In this instance, I've chosen to use the 'viridis' colormap.
"""
plt.scatter (years, pass_exam_computer_science, c = colors, cmap = 'viridis', s=50)
plt.title ("This is yearly report computer science exam ")
plt.xlabel("Years")
plt.ylabel("Passing percantage")
plt.grid()
plt.show()
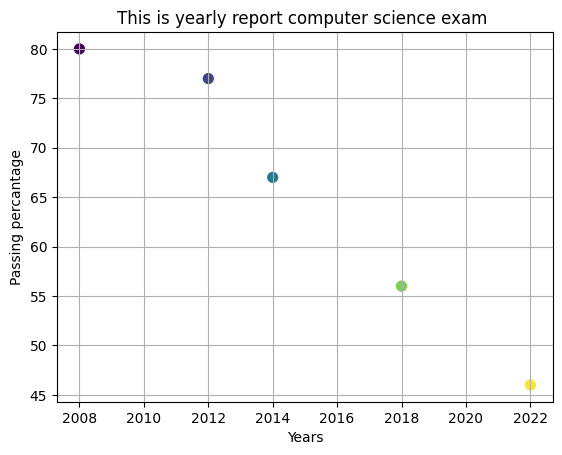
분산형 차트의 도트 크기 변경
개별 도트의 크기를 수정하여 Matplotlib에서 분산형 차트를 향상시키는 방법을 살펴보세요. 이러한 사용자 지정은 특정 데이터 포인트에 주의를 집중시키거나 패턴을 더 효과적으로 강조할 수 있습니다.
# This is a computer science exam passing data
years = np.array([2008, 2012, 2014, 2018, 2022])
pass_exam_computer_science = np.array([80, 77, 67, 56, 46])
"""
The 's' keyword in Matplotlib allows you to adjust the size of the dots
in your scatter plot. Before using it, you'll need to create an array
specifying the desired sizes.
"""
sizes = np.array ([36, 100, 150, 90, 300])
#Now it’s time to pass, your array in the s argument
plt.scatter( years, pass_exam_computer_science, s=sizes)
plt.title ("This is yearly report computer science exam ")
plt.xlabel("Years")
plt.ylabel("Passing percantage")
plt.grid()
plt.show()
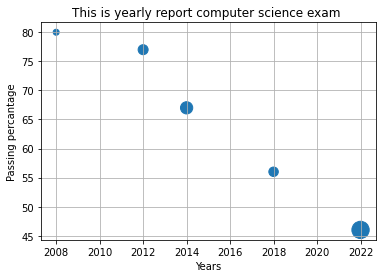
[참고] 컬러맵을 사용하거나 점 크기를 조정할 때 배열 크기가 일치하는지 확인하세요. 이는 x와 y 배열의 길이가 같아야 함을 의미합니다.
first_array = np.array ([1, 2, 3, 4])
secound_array = np.array ([1, 2, 3])
plt.scatter (first_array, secound_array)
# We run this code show -- ValueError: x and y must be the same size
Matplotlib에서 막대 그래프 그리는 방법
Matplotlib를 사용해 막대형 차트를 만드는 단계별 과정을 살펴보세요. 막대형 막대그래프는 범주형 데이터를 비교하고 추세를 시각화하기 위한 훌륭한 도구입니다. Matplotlib Pyplot 모듈에서 bar( ) 함수는 막대 차트{또는 막대 그래프}를 생성하기 위한 핵심 도구입니다.
bank_name = np.array(["Yes Bank", "Axis Bank", "HDFC Bank", "State Bank"])
bank_growth_this_year = np.array([10, 60, 40, 88])
# Specify colors for each bank
colors = np.array(["blue", "red", "black", "skyblue"])
plt.bar(bank_name, bank_growth_this_year, color=colors)
plt.title("Bank growth report in this 2021 Year")
plt.xlabel("Bank Name")
plt.ylabel("Growth percentage")
#plt.grid(True) # Add grid
plt.show()
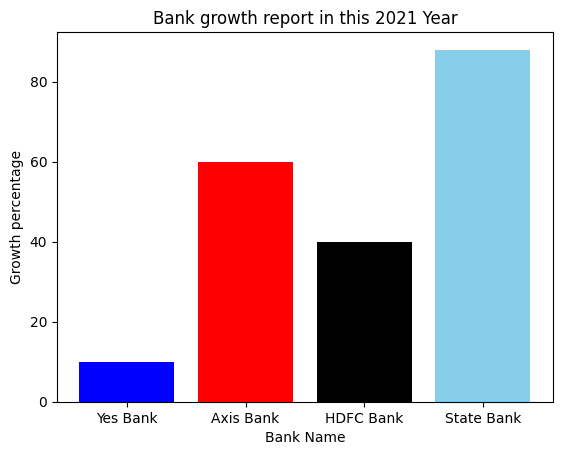
다른 plot을 만들어 봅니다.
# This is a computer science exam passing data
years = np.array([2008, 2012, 2014, 2018, 2022])
pass_exam_computer_science = np.array([80, 77, 67, 56, 46])
sizes = np.array([36, 100, 150, 90, 300])
# Specify colors for each bar
colors = np.array(["skyblue", "gray", "red", "purple", "lightgreen"])
plt.bar(years, pass_exam_computer_science, color=colors)
plt.title("This is yearly report computer science exam ")
plt.xlabel("Years")
plt.ylabel("Passing percentage")
#plt.grid()
plt.show()
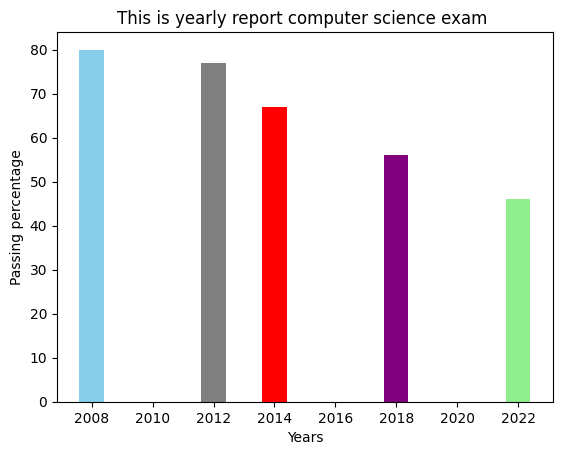
하나 더 만들어 봅니다. 가로 막대 그래프입니다.
# This is a computer science exam passing data
years = np.array([2008, 2012, 2014, 2018, 2022])
pass_exam_computer_science = np.array([80, 77, 67, 56, 46])
sizes = np.array ([36, 100, 150, 90, 300])
#Use barh( ) function to plot the horizontal bar in your data
plt.barh( years, pass_exam_computer_science)
plt.title ("This is yearly report computer science exam ")
plt.xlabel("Years")
plt.ylabel("Passing percantage")
plt.grid()
plt.show()
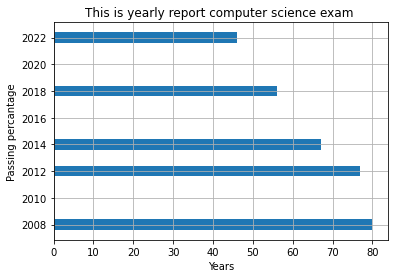
막대 색상 변경하기
bank_name = np.array(["Yes Bank", "Axis Bank", "HDFC Bank", "State Bank"])
bank_growth_this_year = np.array([10, 60, 40, 88])
"""
You have the flexibility to modify the colour of your vertical and
horizontal bars using the `color` keyword. Simply specify the desired colour.
"""
plt.bar(bank_name, bank_growth_this_year, color="lightgreen", width=0.2) # Adjust width as needed
plt.title("Bank growth report in this 2021 Year")
plt.xlabel("Bank Name")
plt.ylabel("Growth percentage")
plt.show()
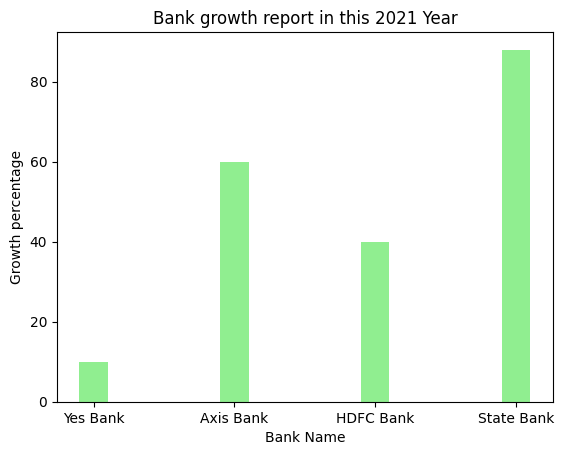
다른 예제를 봅니다.
best_tech_company = np.array(["Apple", "Facebook", "Microsoft", "Amazon", "Google"])
company_growth_this_month = np.array([60, 45, 30, 85, 70])
# Specify colors for each company
colors = np.array(["black", "blue", "green", "orange", "skyblue"])
plt.barh(best_tech_company, company_growth_this_month, color=colors)
plt.title("Company growth this month report")
plt.xlabel("Growth percentage")
plt.ylabel("Company Name")
plt.show()
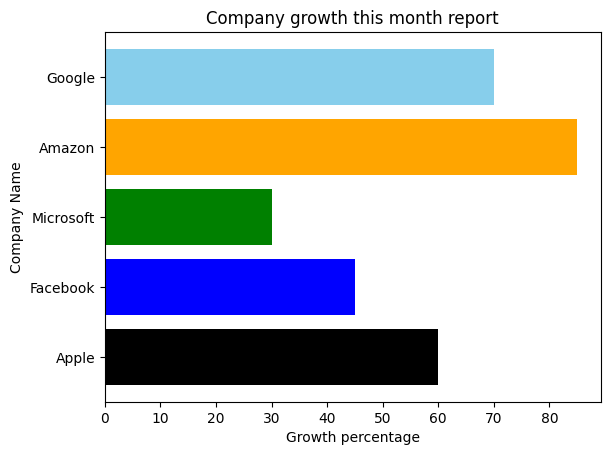
다음은 몇 가지 색상 목록이며, 막대 그래프를 사용해 볼 수 있습니다. 또는 16진수 색상을 사용할 수도 있습니다. 다음은 두 개의 막대 차트를 나란히 그리는 방법의 예시입니다.
# Data for the first plot
bank_name = np.array(["Yes Bank", "Axis Bank", "HDFC Bank", "State Bank"])
bank_growth_this_year = np.array([10, 60, 40, 88])
# Data for the second plot
best_tech_company = np.array(["Apple", "Facebook", "Microsoft", "Amazon", "Google"])
company_growth_this_month = np.array([60, 45, 30, 85, 70])
colors = np.array(["black", "blue", "green", "orange", "skyblue"])
# Create a figure with two subplots
plt.figure(figsize=(12, 6))
# Plot the first subplot
plt.subplot(1, 2, 1) # 1 row, 2 columns, first subplot
plt.bar(bank_name, bank_growth_this_year, color="lightgreen", width=0.2)
plt.title("Bank growth report in this 2021 Year")
plt.xlabel("Bank Name")
plt.ylabel("Growth percentage")
# Plot the second subplot
plt.subplot(1, 2, 2) # 1 row, 2 columns, second subplot
plt.barh(best_tech_company, company_growth_this_month, color=colors)
plt.title("Company growth this month report")
plt.xlabel("Growth percentage")
plt.ylabel("Company Name")
# Adjust layout for better spacing
plt.tight_layout()
# Show the figure
plt.show()
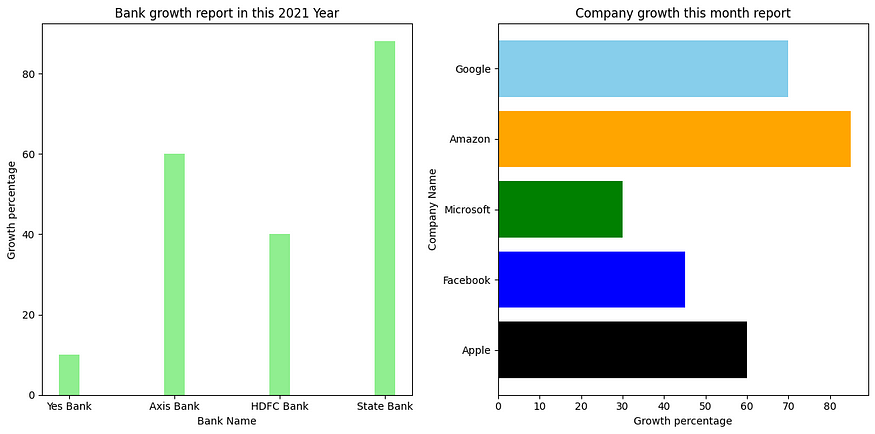
Matplotlib에서 막대 너비 변경하는 방법
이제 막대 너비를 조정하여 좀 더 멋지게 만들어 보겠습니다. 약간의 디자인 마법이 우리의 플롯을 돋보이게 만들 수 있습니다.
best_tech_company = np.array (["Apple", "Facebook", "Microsoft", "Amazon", "Google"])
company_growth_this_month = np.array ([60, 45, 30, 85, 70])
"""
Simply adjust the width of your bars using the 'width' keyword and
set your preferred value. By the way, the default width is 0.8
"""
plt.bar(best_tech_company, company_growth_this_month, width = 0.1, color = "#FAF18E")
plt.title("Company growth this month report")
plt.xlabel("Company Name")
plt.ylabel("Growth percantage")
plt.grid()
plt.show()
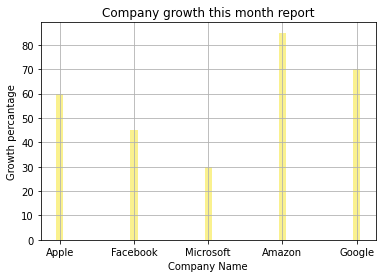
수평 plot을 만들어 봅니다.
best_tech_company = np.array (["Apple", "Facebook", "Microsoft", "Amazon", "Google"])
company_growth_this_month = np.array ([60, 45, 30, 85, 70])
#Vertical time use the width keyword, Horizontal time use height keyword.
plt.barh(best_tech_company, company_growth_this_month, height = 0.1, color = "#FAF18E")
plt.title("Company growth this month report")
plt.xlabel("Company Name")
plt.ylabel("Growth percantage")
plt.grid()
plt.show()
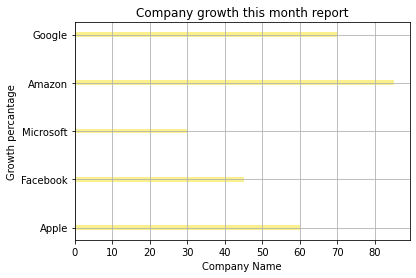
하나의 그림에 함께 나타내 보겠습니다.
# Data for the first plot
best_tech_company = np.array(["Apple", "Facebook", "Microsoft", "Amazon", "Google"])
company_growth_this_month = np.array([60, 45, 30, 85, 70])
# Create a figure with two subplots side by side
plt.figure(figsize=(12, 6))
# Plot the first subplot (vertical bars)
plt.subplot(1, 2, 1) # 1 row, 2 columns, first subplot
plt.bar(best_tech_company, company_growth_this_month, width=0.1, color="#FAF18E")
plt.title("Vertical Bars - Company growth this month report")
plt.xlabel("Company Name")
plt.ylabel("Growth percentage")
plt.grid()
# Plot the second subplot (horizontal bars)
plt.subplot(1, 2, 2) # 1 row, 2 columns, second subplot
plt.barh(best_tech_company, company_growth_this_month, height=0.1, color="#FAF18E")
plt.title("Horizontal Bars - Company growth this month report")
plt.xlabel("Growth percentage")
plt.ylabel("Company Name")
plt.grid()
# Adjust layout for better spacing
plt.tight_layout()
# Show the figure
plt.show()
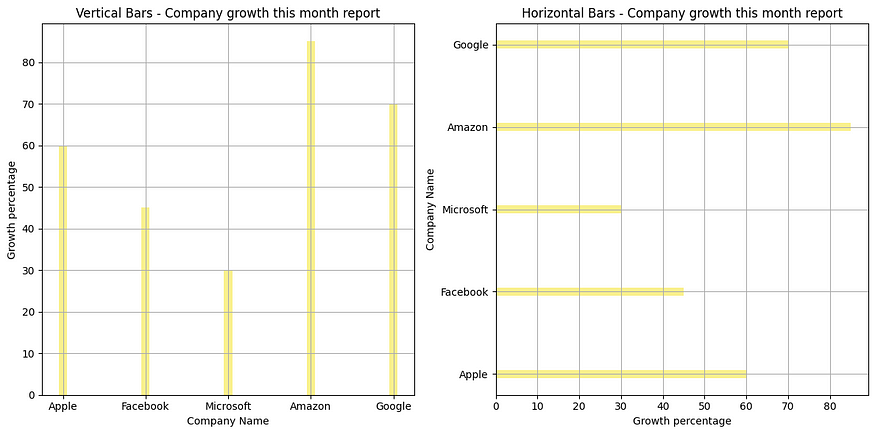
Matplotlib를 사용하여 히스토그램 플롯 만들기
막대형 차트와 마찬가지로 히스토그램은 특정 간격 내의 데이터 포인트의 빈도를 표시하는 데이터의 그래픽 표현입니다. 각 간격은 세로 막대로 표시됩니다. 처음에는 혼란스러워 보일 수 있지만 연습하면 더 명확해집니다. 이를 마스터하기 위한 핵심은 연습입니다.
average_salary_india = np.array([5000, 8000, 9500, 10000, 14500])
companies = ["A", "B", "C", "D", "E"]
plt.bar(companies, average_salary_india, width=0.2, color="lightgreen", edgecolor="black")
# Adjust the width and color as needed
plt.title("Average Salary of Employees in India")
#plt.xlabel("Company")
#plt.ylabel("Average Salary")
plt.grid(axis='y') # Add grid to the y-axis for better readability
plt.show()
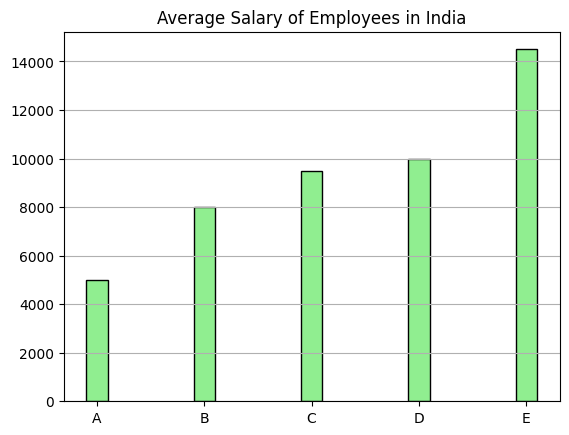
Matplotlib에서 원형 차트 만들기
이 섹션에서는 Python을 사용하여 Matplotlib에서 시각적으로 매력적인 원형 차트를 만드는 방법을 알아봅니다. 원형 차트는 데이터 분포를 나타내는 훌륭한 방법이며 Matplotlib을 사용하면 이를 쉽게 생성할 수 있습니다.
pie_chart_i_learn = np.array([21, 33, 30, 45, 65])
#You can use pie ( ) function, to create pie chart.
plt.pie(pie_chart_i_learn)
plt.show()
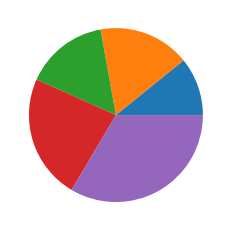
원형 차트에서 데이터의 각 값은 쐐기 모양의 슬라이스로 표시되며, 이러한 슬라이스를 합쳐서 전체 차트를 구성합니다. 모든 차트에는 X축과 Y축이라는 두 가지 중요한 요소가 있습니다. 기본 원형 차트에서 첫 번째 슬라이스는 X축에서 시작하여 시계 반대 방향으로 이동합니다.
company_growth_this_year = np.array([68, 75, 21, 40, 33, 80])
company_name = np.array(["Amazon", "Walmart", "Facebook", "Microsoft", "Google", "Apple"])
# Custom colors for each company
company_colors = {
"Amazon": "skyblue",
"Walmart": "lightgreen",
"Facebook": "orange",
"Microsoft": "red",
"Google": "lightblue",
"Apple": "gray"
}
# Retrieve colors for each company or use a default color
colors = [company_colors.get(company, "gray") for company in company_name]
# Set the figure size
plt.figure(figsize=(8, 8))
plt.pie(company_growth_this_year, labels=company_name, colors=colors, autopct='%1.1f%%', startangle=140)
# Add grid
plt.grid(True, linestyle='--', alpha=0.7)
# Modify title color and font style
plt.title("Company Growth This Year", loc="left", fontdict={'fontsize': 16, 'fontweight': 'bold', 'fontstyle': 'italic', 'color': 'black'})
# Modify company name font style
plt.setp(plt.gca().get_xticklabels(), fontweight='bold', color='black')
plt.show())
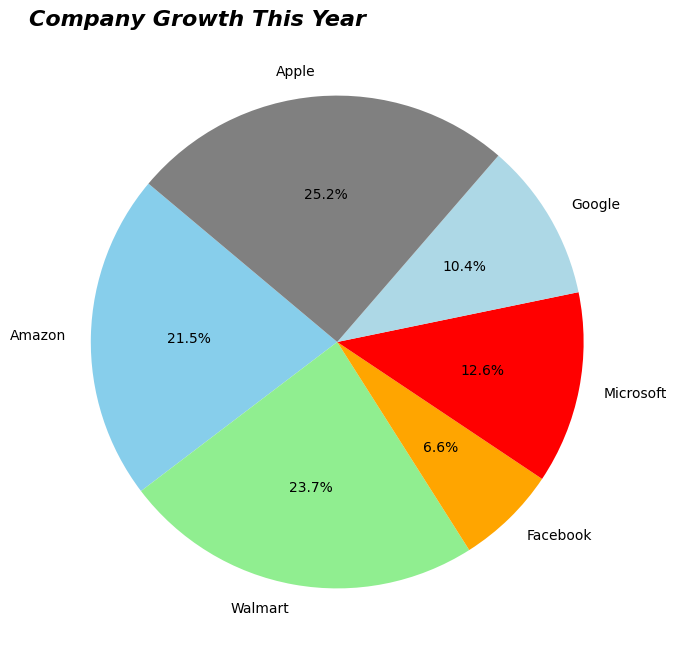
Matplotlib에서 웨지 파이 차트의 각도 변경합니다.
company_growth_this_year = np.array([68, 75, 21, 40, 33, 80])
company_name = np.array(["Amazon", "Walmart", "Facebook", "Microsoft", "Google", "Apple"])
# Custom colors for each company
company_colors = {
"Amazon": "skyblue",
"Walmart": "lightgreen",
"Facebook": "orange",
"Microsoft": "red",
"Google": "lightblue",
"Apple": "gray"
}
# Retrieve colors for each company or use a default color
colors = [company_colors.get(company, "gray") for company in company_name]
# Set the figure size
plt.figure(figsize=(8, 8))
# Change the start angle to 90 degrees
plt.pie(company_growth_this_year, labels=company_name, colors=colors, autopct='%1.1f%%', startangle=90)
# Add grid
plt.grid(True, linestyle='--', alpha=0.7)
# Modify title color and font style
plt.title("Company Growth This Year", loc="left", fontdict={'fontsize': 16, 'fontweight': 'bold', 'fontstyle': 'italic', 'color': 'black'})
# Modify company name font style
plt.setp(plt.gca().get_xticklabels(), fontweight='bold', color='black')
plt.show()
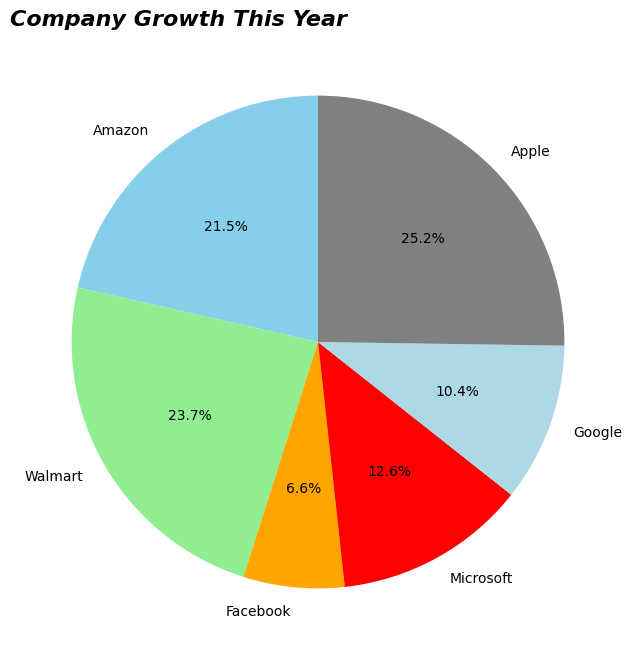
차이점을 발견하셨나요? 두 이미지를 나란히 놓고 살펴보세요.
# Data for the first pie chart
company_growth_this_year_1 = np.array([68, 75, 21, 40, 33, 80])
company_name_1 = np.array(["Amazon", "Walmart", "Facebook", "Microsoft", "Google", "Apple"])
# Custom colors for each company
company_colors_1 = {
"Amazon": "skyblue",
"Walmart": "lightgreen",
"Facebook": "orange",
"Microsoft": "red",
"Google": "lightblue",
"Apple": "gray"
}
# Retrieve colors for each company or use a default color
colors_1 = [company_colors_1.get(company, "gray") for company in company_name_1]
# Data for the second pie chart
company_growth_this_year_2 = np.array([68, 75, 21, 40, 33, 80])
company_name_2 = np.array(["Amazon", "Walmart", "Facebook", "Microsoft", "Google", "Apple"])
# Custom colors for each company
company_colors_2 = {
"Amazon": "skyblue",
"Walmart": "lightgreen",
"Facebook": "orange",
"Microsoft": "red",
"Google": "lightblue",
"Apple": "gray"
}
# Retrieve colors for each company or use a default color
colors_2 = [company_colors_2.get(company, "gray") for company in company_name_2]
# Set up a subplot with 1 row and 2 columns
plt.figure(figsize=(16, 8))
# Plot the first pie chart in the first subplot
plt.subplot(1, 2, 1)
plt.pie(company_growth_this_year_1, labels=company_name_1, colors=colors_1, autopct='%1.1f%%', startangle=140)
plt.grid(True, linestyle='--', alpha=0.7)
plt.title("Company Growth This Year (Chart 1)", loc="left", fontdict={'fontsize': 16, 'fontweight': 'bold', 'fontstyle': 'italic', 'color': 'black'})
plt.setp(plt.gca().get_xticklabels(), fontweight='bold', color='black')
# Plot the second pie chart in the second subplot
plt.subplot(1, 2, 2)
plt.pie(company_growth_this_year_2, labels=company_name_2, colors=colors_2, autopct='%1.1f%%', startangle=90)
plt.grid(True, linestyle='--', alpha=0.7)
plt.title("Company Growth This Year (Chart 2)", loc="left", fontdict={'fontsize': 16, 'fontweight': 'bold', 'fontstyle': 'italic', 'color': 'black'})
plt.setp(plt.gca().get_xticklabels(), fontweight='bold', color='black')
# Adjust layout to prevent overlap
plt.tight_layout()
plt.show()
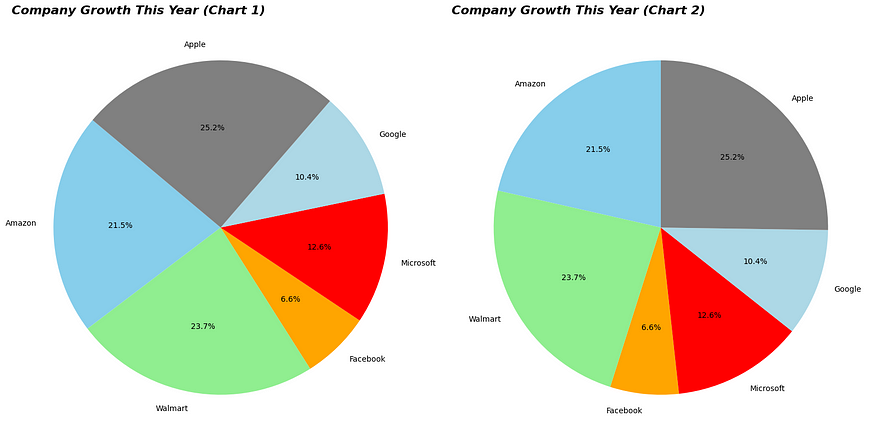
이미 알고 있듯이 기본 시작 각도는 X축 값이 0도입니다. 하지만 startangle 키워드를 사용하여 이 각도를 변경하고 값을 전달할 수 있습니다. 이 경우 각도를 90도로 설정합니다.
파이 차트를 이렇게 표현할 수도 있습니다(Explode Pie Chart).
banks = ["SBI", "Axis Bank", "Yes Bank", "Bank of Baroda", "HDFC Bank"]
sizes = [21, 7, 2, 5, 15]
fig, ax = plt.subplots()
ax.pie(sizes, explode=(0, 0.1, 0.1, 0.1, 0.1), labels=banks, autopct='%1.1f%%',
shadow=True, startangle=90)
ax.axis('equal')
ax.set_title("Top Banks in India by Percentage")
plt.show()
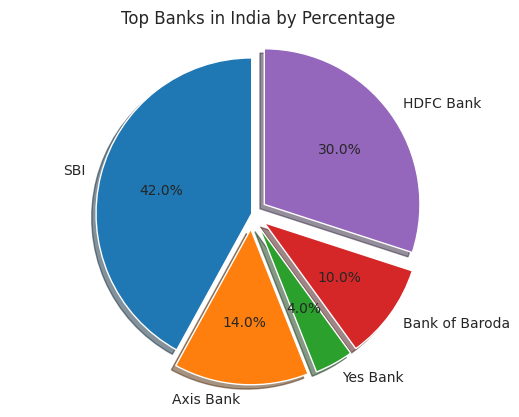
코드를 이해해 봅시다. 플롯을 분해시키려면 explode 매개변수를 사용하고 값을 전달하세요. 예를 들어,
ax.pie(data, explode=(0.1, 0, 0, 0))
Matplotlib에서 원형 차트의 각 웨지 색상 변경하는 코드입니다.
`banks = ["SBI", "Axis Bank", "Yes Bank", "Bank of Baroda", "HDFC Bank"]
sizes = [21, 7, 2, 5, 15]
colors = ['brown', 'lightcoral', 'cyan', 'orange', 'blue']
fig, ax = plt.subplots()
ax.pie(sizes, explode=(0, 0.1, 0.1, 0.1, 0.1), colors=colors,
labels=banks, autopct='%1.1f%%', shadow=True, startangle=90)
ax.axis('equal')
ax.set_title("Top Banks in India by Percentage")
plt.show()
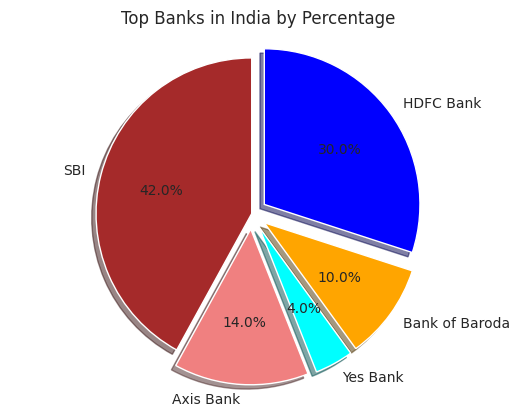
Matplotlib 그림(Plot)을 PNG로 저장하기
방법은 간단합니다. savefig() 함수를 사용하여 이 플롯을 컴퓨터에 저장할 수 있습니다.
`banks = ["SBI", "Axis Bank", "Yes Bank", "Bank of Baroda", "HDFC Bank"]
sizes = [21, 7, 2, 5, 15]
colors = ['brown', 'lightcoral', 'cyan', 'orange', 'blue']
fig, ax = plt.subplots()
patches, texts, autotexts = ax.pie(sizes, explode=(0, 0.1, 0.1, 0.1, 0.1), colors=colors,
labels=banks, autopct='%1.1f%%', shadow=True, startangle=90)
# Add legend
ax.legend(patches, banks, loc="upper left", bbox_to_anchor=(1, 0.5), title="Banks")
# Set equal aspect ratio
ax.axis('equal')
ax.set_title("Top Banks in India by Percentage")
# Save the image
plt.savefig("pie_chart_banks.png", bbox_inches="tight")
# Show the plot
plt.show()
이제 숙제를 하고 더 많이 연습할 시간입니다. 처음에는 모든 것이 생소하고 어려울 수 있지만 연습을 하면 더 쉬워진다는 것을 기억하세요.
'Python' 카테고리의 다른 글
파이썬으로 PDF 양식 데이터 추출 (7) | 2024.03.31 |
---|---|
파이썬으로 QR 코드 생성하는 방법 (5) | 2024.03.30 |
데이터 과학자의 Python 코드 주석 활용법 (8) | 2024.03.15 |
파이썬에서 pdf를 워드(.docx) 파일로 변환하는 방법 (11) | 2024.03.01 |
데이터 과학을 위한 파이썬 무료 강좌 5가지 (8) | 2024.02.25 |