들어가기 전에
Excel 파일이나 워크시트를 병합하는 것은 사용자가 여러 스프레드시트의 데이터를 하나의 통합된 파일로 결합할 수 있게 해주는 중요한 데이터 관리 작업입니다. Python으로 여러 Excel 파일이나 여러 Excel 파일의 특정 워크시트를 단일 파일로 병합하는 방법을 소개합니다. Excel에서 파워 쿼리를 사용하여 파일이나 워크시트를 통합하는 방법에 대한 영상도 있으니 함께 참고하세요.
권현욱(엑셀러) | 아이엑셀러 닷컴 대표 · Microsoft MVP · 엑셀 솔루션 프로바이더 · 작가
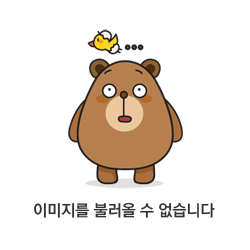
이 글은 아래 기사 내용을 토대로 작성되었습니다만, 필자의 개인 의견이나 추가 자료들이 다수 포함되어 있습니다.
- 원문: Merge Excel Files and Worksheets with Python
- URL: https://medium.com/@alexaae9/merge-excel-files-and-worksheets-with-python-97250104d442
엑셀의 파워 쿼리를 사용해도 파일/워크시트를 통합할 수 있습니다. 오래 전 영상이라 배경 음악이 좀 크게 들릴 수 있으니 감안하고 보세요.ㅎㅎ
참고 영상 1
참고 영상2
Excel 병합을 위한 Python 라이브러리
Python용 Spire.XLS는 Excel 파일을 프로그래밍 방식으로 작업할 수 있는 포괄적인 도구 세트를 제공하는 강력한 라이브러리입니다. 이 라이브러리를 활용하면 Excel 파일을 병합하는 작업을 자동화하여 프로세스를 보다 효율적으로 만들고 오류를 줄일 수 있습니다. 이 라이브러리는 PyPI에서 다음 pip 명령을 사용하여 설치할 수 있습니다.
pip install Spire.Xls
여러 Excel 파일을 단일 파일로 병합하기
Python용 Spire.XLS는 사용자가 한 통합 문서에서 다른 통합 문서로 워크시트를 복사할 수 있는 WorksheetsCollection.AddCopy() 메서드를 제공합니다. 각 Excel 파일에서 워크시트를 검색하여 새 통합 문서에 복사하면 여러 Excel 파일을 단일 파일로 효율적으로 결합할 수 있습니다. 여러 Excel 파일을 병합하는 주요 단계는 다음과 같습니다.
- 병합할 Excel 통합 문서의 파일 경로를 수집합니다.
- 새 통합 문서와 임시 통합 문서를 만듭니다.
- 파일을 반복하여 임시 통합 문서에 로드하고 워크시트를 새 통합 문서에 복사합니다.
- 병합된 통합 문서를 새 파일에 저장합니다.
from spire.xls import *
from spire.xls.common import *
# Put the file paths of the workbooks to be merged into a list
files = []
files.append("C:\\Users\\Administrator\\Desktop\\Input1.xlsx" )
files.append("C:\\Users\\Administrator\\Desktop\\Input2.xlsx")
files.append("C:\\Users\\Administrator\\Desktop\\Input3.xlsx")
# Create a new workbook
newbook = Workbook()
# Clear the default worksheets
newbook.Worksheets.Clear()
# Create a temporary workbook
tempbook = Workbook()
# Loop through the file paths in the list
for file in files:
# Load the workbook specified by the file path into the temporary workbook object
tempbook.LoadFromFile(file)
# Loop through the worksheets in the temporary workbook
for sheet in tempbook.Worksheets:
# Copy the worksheet from the temporary workbook to the new workbook
newbook.Worksheets.AddCopy(sheet, WorksheetCopyType.CopyAll)
# Save the new workbook to a .xlsx file
newbook.SaveToFile("MergeExcelFiles.xlsx", ExcelVersion.Version2016)
# Dispose resources
newbook.Dispose()
tempbook.Dispose()
여러 Excel 파일의 시트를 하나의 파일로 병합하기
서로 다른 Excel 파일의 모든 워크시트를 하나의 파일로 병합하는 대신 결합할 워크시트를 선택적으로 선택할 수 있습니다. 이 접근 방식을 사용하면 데이터 통합 작업을 보다 세분화할 수 있습니다. 핵심 단계는 다음과 같습니다.
- 소스 Excel 파일을 다른 통합 문서 개체에 로드합니다.
- 이 파일에서 원하는 워크시트를 가져옵니다.
- 새 통합 문서를 만듭니다.
- 선택한 워크시트를 새 통합 문서에 추가합니다.
- 새 통합 문서를 Excel 파일에 저장합니다.
from spire.xls import *
from spire.xls.common import *
# Create three temporary workbooks
tempbook_1 = Workbook()
tempbook_2 = Workbook()
tempbook_3 = Workbook()
# Load the source Excel document seperately
tempbook_1.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Input1.xlsx")
tempbook_2.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Input2.xlsx")
tempbook_3.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Input3.xlsx")
# Get the specific worksheets from these files
sheet_1 = tempbook_1.Worksheets.get_Item(0)
sheet_2 = tempbook_1.Worksheets.get_Item(1)
sheet_3 = tempbook_2.Worksheets.get_Item(1)
sheet_4 = tempbook_3.Worksheets.get_Item(2)
# Create a new workbook
newbook = Workbook()
# Clear the default worksheets
newbook.Worksheets.Clear()
# Copy the selected worksheets to the new workbook
newbook.Worksheets.AddCopy(sheet_1, WorksheetCopyType.CopyAll)
newbook.Worksheets.AddCopy(sheet_2, WorksheetCopyType.CopyAll)
newbook.Worksheets.AddCopy(sheet_3, WorksheetCopyType.CopyAll)
newbook.Worksheets.AddCopy(sheet_4, WorksheetCopyType.CopyAll)
# Save the new workbook to a .xlsx file
newbook.SaveToFile("MergeWorksheets.xlsx", ExcelVersion.Version2016)
# Dispose resources
tempbook_1.Dispose()
tempbook_2.Dispose()
tempbook_3.Dispose()
newbook.Dispose()
마치며
파이썬과 Spire.XLS for Python 라이브러리를 활용하여 다양한 Excel 파일의 특정 워크시트를 통합된 Excel 파일로 선택적으로 병합하는 방법에 대해 소개했습니다. Spire.XLS for Python은 이 방법 말고도 다양하게 활용할 수 있습니다. 이와 관련된 보다 자세한 설명은 [여기]를 참고하세요.
'Python' 카테고리의 다른 글
엑셀 데이터와 파이썬을 사용하여 프레젠테이션 자동화하기 (4) | 2025.01.25 |
---|---|
파이썬을 사용하여 Excel에서 그룹화 작업하기 (36) | 2024.12.21 |
파이썬에서 Excel 데이터를 Word 표로 변환하는 2가지 방법 (33) | 2024.12.18 |
파이썬으로 데이터 분석을 배우기 위한 최고의 코스 (20) | 2024.11.15 |
파이썬을 사용하여 Excel 피벗 테이블 다루기 (1) | 2024.11.09 |