들어가기 전에
Excel은 데이터 관리와 계산에 널리 사용되는 반면, Word는 보고서를 비롯한 문서 작성에 장점이 있습니다. Excel 데이터를 Word 표로 변환하면 두 가지 도구의 장점을 결합할 수 있습니다. 파이썬을 사용하여 서식이 있는 Excel 데이터를 Word 표로 원활하게 변환하는 방법을 소개합니다.
권현욱(엑셀러) | 아이엑셀러 닷컴 대표 · Microsoft MVP · 엑셀 솔루션 프로바이더 · 작가
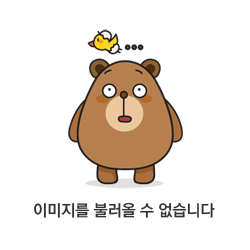
이 글은 아래 기사 내용을 토대로 작성되었습니다만, 필자의 개인 의견이나 추가 자료들이 다수 포함되어 있습니다.
- 원문: Convert Excel Data to Word Table with Formatting in Python (Step by Step Guide)
- URL: https://medium.com/@alice.yang_10652/convert-excel-data-to-word-table-with-formatting-in-python-step-by-step-guide-6767da9b6aa9
Excel 데이터를 Word 표로 변환하는 Python 라이브러리
Excel 데이터를 Word 표로 변환하기 위해 두 가지 Python 라이브러리를 사용하겠습니다: Excel 파일 작업을 위한 Python용 Spire.XLS와 Word 파일 처리를 위한 Python용 Spire.Doc입니다. 다음 명령을 실행하여 파이썬용 Spire.XLS와 파이썬용 Spire.Doc을 설치할 수 있습니다.
pip install spire.xls spire.doc
이 글에서는 Excel 데이터를 Word 표로 변환하는 두 가지 방법을 소개합니다.
- Excel 데이터를 서식과 함께 Word 표로 가져오는 방법
- Excel 파일을 Word 문서에 OLE 개체로 삽입하는 방법
방법 1: Python에서 Excel 데이터를 서식과 함께 Word 표로 가져오기
Excel 데이터를 Word 표로 가져오고 서식을 지정하면 표의 모양을 완벽하게 제어할 수 있습니다. 이 방법을 사용하면 Excel에서 셀 값, 글꼴, 색상, 정렬 및 기타 스타일을 복사하여 최종 Word 표에 원래 서식이 유지되도록 할 수 있습니다. 필요에 따라 사용자 지정 서식을 적용할 수도 있습니다.
(1) Excel 파일 로드하기
먼저, 통합 문서 클래스의 LoadFromFile() 메서드를 사용하여 Excel 파일을 로드합니다. 그런 다음 전송하려는 데이터가 포함된 워크시트를 검색합니다.
workbook = Workbook()
workbook.LoadFromFile("Example.xlsx")
sheet = workbook.Worksheets[0]
(2) Word 문서 만들기 및 표 삽입하기
Document 클래스를 사용하여 새 Word 문서를 만듭니다. 그리고 Table 클래스의 ResetCells 메서드를 사용하여 Excel 워크시트와 동일한 수의 행과 열이 있는 표를 추가합니다.
doc = Document()
section = doc.AddSection()
section.PageSetup.Orientation = PageOrientation.Landscape
table = section.AddTable(True)
table.ResetCells(sheet.LastRow, sheet.LastColumn)
(3) Excel에서 병합된 셀 처리하기
Excel 워크시트에는 병합된 셀이 포함될 수 있으므로 적절하게 관리하는 것이 중요합니다. 이 부분의 로직을 메서드로 캡슐화했습니다.
merge_cells(sheet, table)
다음은 merge_cells 메서드의 구현입니다. 이 메서드에서는 워크시트 클래스의 HasMergedCells 속성을 통해 Excel 시트에 병합된 셀이 있는지 확인합니다. 병합된 셀이 발견되면 Table 클래스의 ApplyHorizongtalMerge 및 ApplyVerticalMerge 메서드를 통해 Word 테이블에서 해당 셀을 병합합니다.
def merge_cells(sheet, table):
if sheet.HasMergedCells:
# Get all merged cell ranges from the Excel worksheet
ranges = sheet.MergedCells
for merged_range in ranges:
# Extract the starting row, column, and the number of rows and columns in the merged range
start_row = merged_range.Row
start_column = merged_range.Column
row_count = merged_range.RowCount
column_count = merged_range.ColumnCount
# Apply both horizontal and vertical merging if multiple rows and columns are merged
if row_count > 1 and column_count > 1:
# Apply horizontal merging for each row within the merged range
for j in range(start_row, start_row + row_count):
table.ApplyHorizontalMerge(j - 1, start_column - 1, start_column - 1 + column_count - 1)
# Apply vertical merging across the merged rows
table.ApplyVerticalMerge(start_column - 1, start_row - 1, start_row - 1 + row_count - 1)
# Apply vertical merging for multi-row merged cells
elif row_count > 1:
table.ApplyVerticalMerge(start_column - 1, start_row - 1, start_row - 1 + row_count - 1)
# Apply horizontal merging for multi-column merged cells
elif column_count > 1:
table.ApplyHorizontalMerge(start_row - 1, start_column - 1, start_column - 1 + column_count - 1)
(4) 워드 표를 Excel 데이터로 채우고 Excel 스타일을 워드 표에 복사
Excel 시트의 각 행과 열을 반복하여 Excel 셀의 데이터와 스타일을 Word 표로 복사합니다.
for r in range(1, sheet.LastRow + 1):
# Set row height in the Word table to match the row height in the Excel sheet
table.Rows[r - 1].Height = float(sheet.Rows[r - 1].RowHeight)
for c in range(1, sheet.LastColumn + 1):
# Get the Excel cell at the current row and column
x_cell = sheet.Range[r, c]
# Get the corresponding Word table cell
w_cell = table.Rows[r - 1].Cells[c - 1]
# Insert the text from the Excel cell into the Word table cell
text_range = w_cell.AddParagraph().AppendText(x_cell.DisplayedText)
# Copy the style (font, color, alignment, etc.) from the Excel cell to the Word cell
copy_style(text_range, x_cell, w_cell)
다음은 copy_style 메서드의 구현입니다. 이 메서드에서는 글꼴 속성 및 스타일(배경색 및 정렬)을 Excel 셀에서 Word 표 셀로 복사합니다.
def copy_style(w_text_range, x_cell, w_cell):
# Copy font formatting (text color, font size, name, and style) from Excel cell to Word text range
w_text_range.CharacterFormat.TextColor = Color.FromRgb(x_cell.Style.Font.Color.R, x_cell.Style.Font.Color.G, x_cell.Style.Font.Color.B)
w_text_range.CharacterFormat.FontSize = float(x_cell.Style.Font.Size)
w_text_range.CharacterFormat.FontName = x_cell.Style.Font.FontName
w_text_range.CharacterFormat.Bold = x_cell.Style.Font.IsBold
w_text_range.CharacterFormat.Italic = x_cell.Style.Font.IsItalic
# Copy background color from Excel cell to Word cell
if x_cell.Style.FillPattern is not ExcelPatternType.none:
w_cell.CellFormat.BackColor = Color.FromRgb(x_cell.Style.Color.R, x_cell.Style.Color.G, x_cell.Style.Color.B)
# Copy horizontal alignment from Excel cell to Word paragraph
if x_cell.HorizontalAlignment == HorizontalAlignType.Left:
w_text_range.OwnerParagraph.Format.HorizontalAlignment = HorizontalAlignment.Left
elif x_cell.HorizontalAlignment == HorizontalAlignType.Center:
w_text_range.OwnerParagraph.Format.HorizontalAlignment = HorizontalAlignment.Center
elif x_cell.HorizontalAlignment == HorizontalAlignType.Right:
w_text_range.OwnerParagraph.Format.HorizontalAlignment = HorizontalAlignment.Right
# Copy vertical alignment from Excel cell to Word cell
if x_cell.VerticalAlignment == VerticalAlignType.Bottom:
w_cell.CellFormat.VerticalAlignment = VerticalAlignment.Bottom
elif x_cell.VerticalAlignment == VerticalAlignType.Center:
w_cell.CellFormat.VerticalAlignment = VerticalAlignment.Middle
elif x_cell.VerticalAlignment == VerticalAlignType.Top:
w_cell.CellFormat.VerticalAlignment = VerticalAlignment.Top
(5) 생성된 Word 문서 저장
마지막으로, Document 클래스의 SaveToFile 메서드를 사용하여 생성된 Word 문서를 저장합니다.
doc.SaveToFile("ExcelToWordTable.docx", FileFormat.Docx2016)
전체 코드는 다음과 같습니다.
from spire.xls import *
from spire.doc import *
# Function to apply cell merging from Excel sheet to Word table
# It checks if the Excel worksheet has merged cells, then merges the corresponding cells in the Word table.
def merge_cells(sheet, table):
if sheet.HasMergedCells:
# Get all merged cell ranges from the Excel worksheet
ranges = sheet.MergedCells
for merged_range in ranges:
# Extract the starting row, column, and the number of rows and columns in the merged range
start_row = merged_range.Row
start_column = merged_range.Column
row_count = merged_range.RowCount
column_count = merged_range.ColumnCount
# Apply both horizontal and vertical merging if multiple rows and columns are merged
if row_count > 1 and column_count > 1:
# Apply horizontal merging for each row within the merged range
for j in range(start_row, start_row + row_count):
table.ApplyHorizontalMerge(j - 1, start_column - 1, start_column - 1 + column_count - 1)
# Apply vertical merging across the merged rows
table.ApplyVerticalMerge(start_column - 1, start_row - 1, start_row - 1 + row_count - 1)
# Apply vertical merging for multi-row merged cells
elif row_count > 1:
table.ApplyVerticalMerge(start_column - 1, start_row - 1, start_row - 1 + row_count - 1)
# Apply horizontal merging for multi-column merged cells
elif column_count > 1:
table.ApplyHorizontalMerge(start_row - 1, start_column - 1, start_column - 1 + column_count - 1)
# Function to copy formatting from Excel cells to Word table cells
# It replicates font properties, background color, and alignment (horizontal and vertical) from Excel to Word
def copy_style(w_text_range, x_cell, w_cell):
# Copy font formatting (color, size, font name, bold, italic) from Excel cell to Word text range
w_text_range.CharacterFormat.TextColor = Color.FromRgb(x_cell.Style.Font.Color.R, x_cell.Style.Font.Color.G, x_cell.Style.Font.Color.B)
w_text_range.CharacterFormat.FontSize = float(x_cell.Style.Font.Size)
w_text_range.CharacterFormat.FontName = x_cell.Style.Font.FontName
w_text_range.CharacterFormat.Bold = x_cell.Style.Font.IsBold
w_text_range.CharacterFormat.Italic = x_cell.Style.Font.IsItalic
# Copy background color from Excel cell to Word cell
if x_cell.Style.FillPattern is not ExcelPatternType.none:
w_cell.CellFormat.BackColor = Color.FromRgb(x_cell.Style.Color.R, x_cell.Style.Color.G, x_cell.Style.Color.B)
# Copy horizontal alignment from Excel cell to Word paragraph
if x_cell.HorizontalAlignment == HorizontalAlignType.Left:
w_text_range.OwnerParagraph.Format.HorizontalAlignment = HorizontalAlignment.Left
elif x_cell.HorizontalAlignment == HorizontalAlignType.Center:
w_text_range.OwnerParagraph.Format.HorizontalAlignment = HorizontalAlignment.Center
elif x_cell.HorizontalAlignment == HorizontalAlignType.Right:
w_text_range.OwnerParagraph.Format.HorizontalAlignment = HorizontalAlignment.Right
# Copy vertical alignment from Excel cell to Word cell
if x_cell.VerticalAlignment == VerticalAlignType.Bottom:
w_cell.CellFormat.VerticalAlignment = VerticalAlignment.Bottom
elif x_cell.VerticalAlignment == VerticalAlignType.Center:
w_cell.CellFormat.VerticalAlignment = VerticalAlignment.Middle
elif x_cell.VerticalAlignment == VerticalAlignType.Top:
w_cell.CellFormat.VerticalAlignment = VerticalAlignment.Top
# Load the Excel file into a Workbook object
workbook = Workbook()
workbook.LoadFromFile("Example.xlsx")
# Retrieve the first worksheet from the workbook
sheet = workbook.Worksheets[0]
doc = Document()
section = doc.AddSection()
section.PageSetup.Orientation = PageOrientation.Landscape
table = section.AddTable(True)
table.ResetCells(sheet.LastRow, sheet.LastColumn)
merge_cells(sheet, table)
for r in range(1, sheet.LastRow + 1):
# Set row height in the Word table to match the row height in the Excel sheet
table.Rows[r - 1].Height = float(sheet.Rows[r - 1].RowHeight)
for c in range(1, sheet.LastColumn + 1):
x_cell = sheet.Range[r, c]
w_cell = table.Rows[r - 1].Cells[c - 1]
text_range = w_cell.AddParagraph().AppendText(x_cell.DisplayedText)
copy_style(text_range, x_cell, w_cell)
doc.SaveToFile("ExcelToWordTable.docx", FileFormat.Docx2016)
doc.Close()
workbook.Dispose()
방법 2: Word에 Excel을 OLE 개체로 삽입하기
Excel 데이터를 OLE 개체로 Word에 삽입할 수도 있습니다. 이 방법은 전체 Excel 파일을 Word 문서에 삽입하여 기능을 유지하고 사용자가 삽입된 개체를 두 번 클릭하여 Excel 데이터를 열고 편집할 수 있도록 합니다.
(1) Excel 파일 로드하기
통합 문서 클래스의 LoadFromFile 메서드를 사용하여 Excel 파일을 로드합니다. 그런 다음 전송하려는 데이터가 포함된 워크시트를 검색합니다.
workbook = Workbook()
workbook.LoadFromFile("Example.xlsx")
sheet = workbook.Worksheets[0]
(2) 워크시트를 이미지로 변환하기
워크시트 클래스의 ToImage 메서드를 사용하여 워크시트를 이미지로 저장합니다. 그러면 이 이미지가 Word 문서에서 Excel OLE 개체의 아이콘으로 사용됩니다.
image = sheet.ToImage(1, 1, sheet.LastRow, sheet.LastColumn)
image.Save("ExcelIcon.png")
(3) Word 문서 만들기
Document 클래스를 사용하여 Word 문서를 만듭니다.
doc = Document()
section = doc.AddSection()
section.PageSetup.Margins.All = 72.0
section.PageSetup.Orientation = PageOrientation.Landscape
(4) 변환된 이미지 로드
저장한 이미지를 DocPicture 객체에 로드합니다.
picture = DocPicture(doc)
picture.LoadImage("ExcelIcon.png")
picture.Width = 500.0
picture.Height = 300.0
(5) Word 문서에 Excel 파일을 OLE로 삽입
Word 문서에 단락을 추가하고 로드된 이미지가 아이콘으로 있는 OLE 개체로 Excel 파일을 단락에 추가합니다.
para = section.AddParagraph()
para.AppendOleObject("Example.xlsx", picture, OleObjectType.ExcelWorksheet)
(6) Word 문서 저장
생성된 Word 문서를 Document 클래스의 SaveToFile 메서드를 사용하여 저장합니다.
doc.SaveToFile("InsertExcelIntoWordAsOLE.docx", FileFormat.Docx2013)
전체 코드는 다음과 같습니다.
from spire.xls import *
from spire.doc import *
# Load an Excel file into a Workbook object
workbook = Workbook()
workbook.LoadFromFile("Example.xlsx")
# Retrieve the first worksheet from the workbook
sheet = workbook.Worksheets[0]
# Set the worksheet margins to zero (optional, if not set, the image will have blank margins)
sheet.PageSetup.TopMargin = 0.0
sheet.PageSetup.BottomMargin = 0.0
sheet.PageSetup.LeftMargin = 0.0
sheet.PageSetup.RightMargin = 0.0
# Convert the worksheet to an image
# This image will be used as the icon for the Excel OLE object in the Word document
image = sheet.ToImage(1, 1, sheet.LastRow, sheet.LastColumn)
image.Save("ExcelIcon.png")
# Create a Word document
doc = Document()
# Add a section
section = doc.AddSection()
# Set page margins
section.PageSetup.Margins.All = 72.0
# Set page orientation
section.PageSetup.Orientation = PageOrientation.Landscape
# Load the previously saved image into a DocPicture object
picture = DocPicture(doc)
picture.LoadImage("ExcelIcon.png")
# Set the width and height of the image
picture.Width = 500.0
picture.Height = 300.0
# Add a paragraph to the section
para = section.AddParagraph()
# Append the Excel file to the paragraph as an OLE object using the loaded image as an icon
para.AppendOleObject("Example.xlsx", picture, OleObjectType.ExcelWorksheet)
# Save the Word document with the embedded Excel OLE object
doc.SaveToFile("InsertExcelIntoWordAsOLE.docx", FileFormat.Docx2013)
doc.Close()
workbook.Dispose()
마치며
Python을 사용하여 서식을 유지하면서 Excel 데이터를 Word 데이터로 변환하는 두 가지 방법에 대해 소개했습니다. 자신의 상황에 적합한 것을 선택해서 사용하시기 바랍니다.
'Python' 카테고리의 다른 글
Excel 파일과 워크시트 병합하기 ㅡ 파이썬 + 엑셀 파워 쿼리 (4) | 2025.01.11 |
---|---|
파이썬을 사용하여 Excel에서 그룹화 작업하기 (36) | 2024.12.21 |
파이썬으로 데이터 분석을 배우기 위한 최고의 코스 (20) | 2024.11.15 |
파이썬을 사용하여 Excel 피벗 테이블 다루기 (1) | 2024.11.09 |
파이썬에서 PDF에 디지털 서명을 추가하는 6가지 방법 (3) | 2024.11.03 |