들어가기 전에
데이터 정렬은 데이터 분석에서 기본적인 작업입니다. 이를 통해 데이터를 의미 있는 방식으로 구성하여 패턴, 추세 및 통찰력을 더 쉽게 식별할 수 있습니다. 무료 Python 라이브러리를 활용하여 Excel에서 데이터를 정렬하는 방법에 대해 소개합니다.
권현욱(엑셀러) | 아이엑셀러 닷컴 대표 · Microsoft MVP · 엑셀 솔루션 프로바이더 · 작가
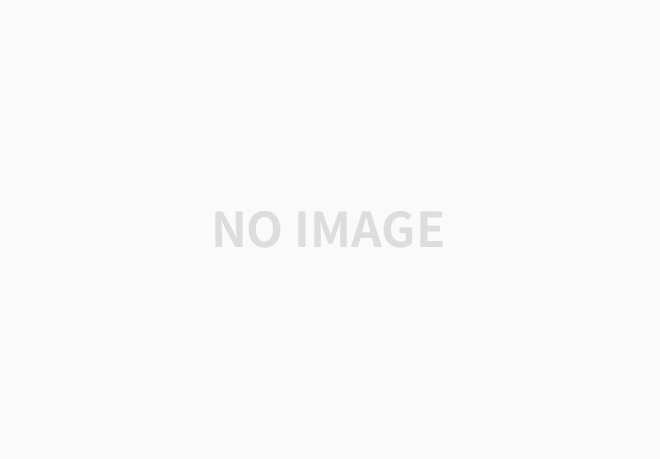
이 글은 아래 기사 내용을 토대로 작성되었습니다만, 필자의 개인 의견이나 추가 자료들이 다수 포함되어 있습니다.
- 원문: Sorting Data in Excel with Python: Techniques for Beginners and Experts
- URL: https://python.plainenglish.io/sorting-data-in-excel-with-python-techniques-for-beginners-and-experts-769c3dd38928
Excel 데이터 정렬에 필요한 파이썬 라이브러리
Python용 무료 Spire.XLS는 Excel 파일을 프로그래밍 방식으로 조작할 수 있는 다용도 라이브러리입니다. 데이터 읽기, 쓰기, 정렬 등 다양한 기능을 지원합니다. Free Spire.XLS를 시작하려면 터미널에서 다음 명령을 실행하여 PyPi를 통해 설치할 수 있습니다.
pip install Spire.Xls.Free
단일 열 데이터 정렬
단일 열에서 데이터를 정렬하는 것은 정보를 효율적으로 정리할 수 있는 Excel의 기본 기술입니다. 이러한 방식으로 정렬하면 데이터를 오름차순 또는 내림차순으로 빠르게 정렬하여 분석하고 해석하기 쉽게 만들 수 있습니다.
Python용 무료 Spire.XLS에는 Workbook.DataSorter.SortColumns.Add(int key, SortComparisonType sortComparisonType, OrderBy orderBy)메서드가 포함되어 있습니다. 이를 통해 특정 열을 선택하고, 비교 유형을 정의하고, 정렬 순서(오름차순 또는 내림차순)를 지정하여 정렬 기준을 설정할 수 있습니다. 정의된 범위에서 정렬을 수행하려면 Workbook.DataSorter.Sort메서드를 사용합니다.
한 가지 주의해야 할 점은 셀 범위에 수식이 포함된 경우 Excel에서 수식을 정적 값이 아닌 동적 값으로 취급하므로 데이터를 직접 정렬할 수 없다는 것입니다. 수식이 있는 셀에서 직접 정렬하면 참조에 따라 수식이 변경될 수 있으므로 예기치 않은 결과가 발생할 수 있습니다. 제대로 정렬하려면 다음과 같이 하는 것이 좋습니다.
- 값 검색: 수식에서 계산된 값을 추출합니다.
- 수식 지우기: 셀의 수식을 정적 값으로 바꿉니다.
- 데이터 정렬: 정적 값에 대한 정렬 작업을 수행합니다.
다음 코드는 수식을 정적 값으로 변환하고 Excel의 특정 열에서 정렬을 수행하는 방법을 보여 줍니다.
from spire.xls.common import *
from spire.xls import *
# Create a Workbook instance
workbook = Workbook()
# Load an Excel file
workbook.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Input.xlsx")
# Get the first worksheet
worksheet = workbook.Worksheets[0]
# Get the cell range (a single column) where you want to sort data
cellRange = worksheet["G1:G13"]
# Iterate through the cells in the range
for cell in cellRange:
# Determine whether the cell contain formula
if (cell.HasFormula):
# If yes, get the formula value in the cell
value = cell.FormulaValue
# Clear cell content
cell.Clear(ExcelClearOptions.ClearContent)
# Fill the formula value into the cell
cell.Value = value
# Sort values in the specified column in descending order
workbook.DataSorter.SortColumns.Add(6, SortComparsionType.Values, OrderBy.Descending)
# Sort in the specified cell range
workbook.DataSorter.Sort(cellRange)
# Save the result file
workbook.SaveToFile("output/SortByColumn.xlsx", ExcelVersion.Version2016)
# Dispose resources
workbook.Dispose()
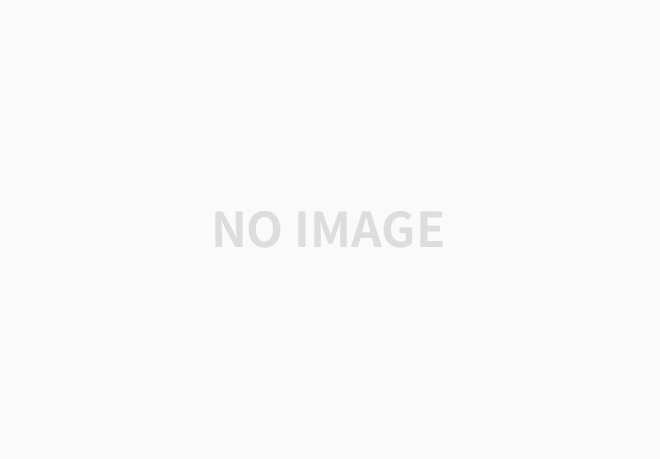
여러 열 데이터 정렬
복잡한 데이터 집합을 다룰 때 여러 열에 걸쳐 정렬하면 데이터 분석을 크게 향상시킬 수 있습니다. 여러 열에 동시에 정렬 기준을 적용하면 서로 다른 데이터 요소 간의 관계를 반영하는 구조화된 보기를 만들어 분석의 통찰력을 높일 수 있습니다.
여러 열에 걸쳐 데이터를 정렬하는 것은 단일 열을 정렬하는 것과 유사합니다. 주요 차이점은 Workbook.DataSorter.Sort를 사용할 때 CellRange 매개 변수가 하나가 아닌 여러 열을 지정한다는 것입니다. 다음 코드는 Python을 사용하여 Excel에서 여러 열에 걸쳐 데이터를 정렬하는 방법을 보여줍니다.
from spire.xls.common import *
from spire.xls import *
# Create a Workbook instance
workbook = Workbook()
# Load an Excel file
workbook.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Input.xlsx")
# Get the first worksheet
worksheet = workbook.Worksheets[0]
# Get the cell range (multiple columns) where you want to sort data
cellRange = worksheet["A1:G13"]
# Iterate through the cells in the range
for cell in cellRange:
# Determine whether the cell contain formula
if (cell.HasFormula):
# If yes, get the formula value in the cell
value = cell.FormulaValue
# Clear cell content
cell.Clear(ExcelClearOptions.ClearContent)
# Fill the formula value into the cell
cell.Value = value
# Sort values in the specified column in descending order
workbook.DataSorter.SortColumns.Add(6, SortComparsionType.Values, OrderBy.Descending)
# Sort in the specified cell range
workbook.DataSorter.Sort(cellRange)
# Save the result file
workbook.SaveToFile("output/SortByColumns.xlsx", ExcelVersion.Version2016)
# Dispose resources
workbook.Dispose()
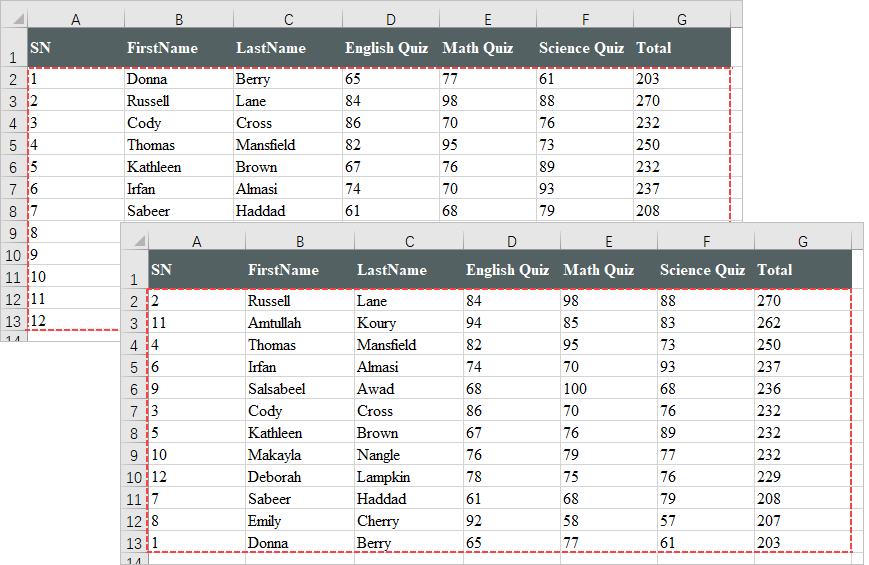
사용자 지정 목록을 사용하여 데이터 정렬
사용자 지정 목록을 사용하여 데이터를 정렬하면 필요에 맞는 특정 순서로 데이터를 유연하게 정렬할 수 있습니다. 고유한 카테고리 순서나 특정 배열을 염두에 두고 있든 이 기능을 사용하면 데이터의 맥락을 유지하면서 원하는 대로 정렬할 수 있습니다.
이를 위해 값을 정렬할 순서를 지정하는 목록을 만들 수 있습니다. 여기서 값은 문자열로 사용자 지정 정렬 순서를 나타냅니다. 그런 다음 Workbook.DataSorter.SortColumns.Add(int key, OrderBy orderBy)메서드를 사용하여 정렬 기준을 설정합니다. 마지막으로, 지정된 셀 범위를 정렬하기 위해 Workbook.DataSorter.Sort메서드를 호출합니다.
from spire.xls.common import *
from spire.xls import *
# Create a Workbook instance
workbook = Workbook()
# Load an Excel file
workbook.LoadFromFile("C:\\Users\\Administrator\\Desktop\\Input.xlsx")
# Get the first worksheet
worksheet = workbook.Worksheets[0]
# Get the cell range where you want to sort data
cellRange = worksheet["A1:G13"]
# Create a custom sort list
customList = ["6", "5", "4", "3", "2", "1", "12", "11", "10", "9", "8" ,"7"]
# Sort a specified column using the custom list
workbook.DataSorter.SortColumns.Add(0, customList )
# Sort in the specified cell range
workbook.DataSorter.Sort(cellRange)
# Save the result file
workbook.SaveToFile("output/CustomSortList.xlsx", ExcelVersion.Version2016)
# Dispose resources
workbook.Dispose()
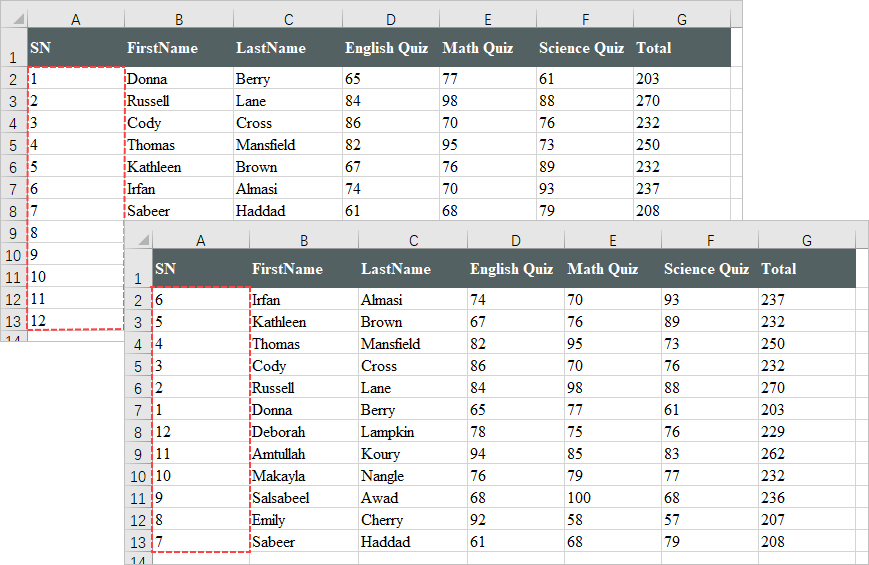
마치며
Python을 사용하여 Excel에서 단일 열, 여러 열, 사용자 지정 목록을 정렬하는 방법을 살펴보았습니다. VBA를 사용하면 더욱 다양한 방식으로 정렬 작업을 수행할 수 있습니다.
'Python' 카테고리의 다른 글
파이썬으로 Excel에서 머리글과 바닥글 제어하는 방법 (5) | 2025.04.02 |
---|---|
Excel의 기능을 확장하는 5가지 파이썬 라이브러리 (3) | 2025.03.09 |
파이썬을 사용하여 Excel에서 테이블 생성/업데이트/제거하기 (3) | 2025.03.08 |
엑셀 데이터와 파이썬을 사용하여 프레젠테이션 자동화하기 (4) | 2025.01.25 |
Excel 파일과 워크시트 병합하기 ㅡ 파이썬 + 엑셀 파워 쿼리 (4) | 2025.01.11 |