들어가기 전에
메일 병합(Mail merge)은 사용자가 템플릿과 데이터 소스를 결합하여 개인화된 문서를 만들 수 있는 Microsoft Word의 강력한 기능입니다. 이 기능은 특히 개별 수신자를 위해 사용자 지정 편지, 레이블, 봉투 및 이메일을 생성하는 데 유용합니다. 파이썬을 사용하여 Word에서 메일 병합을 수행하는 방법을 소개합니다.
권현욱(엑셀러) | 아이엑셀러 닷컴 대표 · Microsoft MVP · 엑셀 솔루션 프로바이더 · 작가
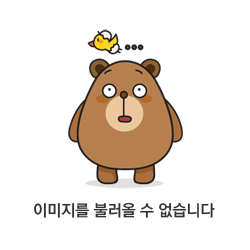
※ 이 글은 아래 기사 내용을 토대로 작성되었습니다만, 필자의 개인 의견이나 추가 자료들이 다수 포함되어 있습니다.
- 원문: Perform Mail Merge in Word with Python: A Comprehensive Guide
- URL: https://medium.com/@alice.yang_10652/perform-mail-merge-in-word-with-python-a-comprehensive-guide-6698341fecbc
편지 병합을 수행하기 위한 파이썬 라이브러리 설치
Python으로 Word에서 편지 병합을 수행하려면 Python용 Spire.Doc 라이브러리를 사용합니다. 이 라이브러리는 Word 문서를 조작하고 프로그래밍 방식으로 편지 병합을 실행하기 위한 강력한 기능을 제공합니다. 터미널에서 다음 명령을 실행하여 PyPI에서 Python용 Spire.Doc을 설치할 수 있습니다.
pip install Spire.Doc
설치에 대한 자세한 내용은 [여기]에서 확인할 수 있습니다.
메일 병합 템플릿 만들기
메일 병합 템플릿은 여러 수신자를 위한 개인화된 커뮤니케이션을 만드는 데 사용되는 미리 디자인된 문서입니다. 이 템플릿에는 모든 수신자에게 동일하게 유지되는 정적 콘텐츠와 데이터 소스의 개인화된 정보로 대체되는 병합 필드라고 하는 자리 표시자가 포함되어 있는 경우가 많습니다.
MS Word를 사용하여 수동으로 편지 병합 템플릿을 만들거나 Python을 사용하여 이 절차를 자동화할 수 있습니다. 다음은 MS Word에서 병합 필드가 있는 템플릿을 만드는 단계입니다.
- Word 문서를 엽니다.
- 정적 콘텐츠(static content)를 삽입할 위치를 클릭합니다.
- 필요에 따라 정적 콘텐츠(텍스트, 이미지 등)를 입력합니다.
- 병합 필드를 삽입할 위치를 클릭합니다.
- 삽입 메뉴로 이동하여 빠른 부분을 선택한 다음 드롭다운 목록에서 필드...를 선택하여 필드 대화 상자를 엽니다.
- 필드 이름 목록에서 병합 필드를 선택합니다.
- 필드 이름 텍스트 상자에 병합 필드의 이름을 입력하고 확인을 클릭합니다.
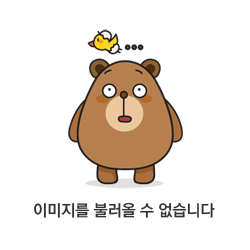
Python을 사용하여 편지 병합 템플릿 생성을 자동화할 수도 있습니다. 다음은 Spire.Doc을 사용하여 프로그래밍 방식으로 편지 병합 템플릿을 만드는 방법의 예입니다.
from spire.doc import *
from spire.doc.common import *
# Create a new document
template = Document()
# Add a section to the document
section = template.AddSection()
# Set the page margins for the section
section.PageSetup.Margins.All = 72 # 1 inch margins
# Add paragraphs with merge fields
paragraph1 = section.AddParagraph()
paragraph1.AppendField("CompanyName", FieldType.FieldMergeField)
paragraph2 = section.AddParagraph()
paragraph2.AppendField("CompanyAddress", FieldType.FieldMergeField)
# Start a new line
paragraph2.AppendBreak(BreakType.LineBreak)
# Add paragraphs with text and merge fields
paragraph3 = section.AddParagraph()
paragraph3.AppendText("Dear ")
paragraph3.AppendField("FirstName", FieldType.FieldMergeField)
paragraph3.AppendText(" ")
paragraph3.AppendField("LastName", FieldType.FieldMergeField)
paragraph3.AppendText(",")
paragraph3.AppendBreak(BreakType.LineBreak)
paragraph4 = section.AddParagraph()
paragraph4.AppendText("We are pleased to inform you about our upcoming conference. It will take place at the following location:")
paragraph4.AppendBreak(BreakType.LineBreak)
paragraph4.AppendText("Event Location: ")
paragraph4.AppendField("EventLocation", FieldType.FieldMergeField)
paragraph4.AppendBreak(BreakType.LineBreak)
paragraph4.AppendText("Event Date: ")
paragraph4.AppendField("EventDate", FieldType.FieldMergeField)
paragraph4.AppendBreak(BreakType.LineBreak)
paragraph5 = section.AddParagraph()
paragraph5.AppendText("Please confirm your attendance by replying to this email.")
paragraph5.AppendBreak(BreakType.LineBreak)
paragraph6 = section.AddParagraph()
paragraph6.AppendText("Sincerely,")
paragraph6.AppendBreak(BreakType.LineBreak)
paragraph6.AppendField("SenderName", FieldType.FieldMergeField)
paragraph6.AppendBreak(BreakType.LineBreak)
paragraph6.AppendField("SenderPosition", FieldType.FieldMergeField)
# Create a paragraph style
para_style = ParagraphStyle(template)
para_style.Name = "ParaStyle"
para_style.ParagraphFormat.LineSpacingRule = LineSpacingRule.Multiple
para_style.ParagraphFormat.LineSpacing = 12
para_style.CharacterFormat.FontName = "Arial"
para_style.CharacterFormat.FontSize = 12
template.Styles.Add(para_style)
# Apply the style to all paragraphs in the section
for i in range(section.Paragraphs.Count):
para = section.Paragraphs[i]
para.ApplyStyle(para_style.Name)
# Save the document as a .docx file
template.SaveToFile("MailMergeTemplate.docx", FileFormat.Docx2016)
# Or you can save the document in other Word formats like .doc
# template.SaveToFile("MailMergeTemplate.doc", FileFormat.Doc)
# Close the document
template.Close()
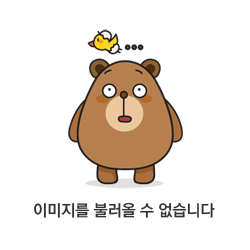
Python용 Spire.Doc의 Document.MailMerge.Execute() 메서드는 편지 병합 템플릿의 병합 필드를 데이터 소스의 데이터로 채우는 데 사용됩니다. 이 메서드에는 두 개의 매개변수가 필요합니다.
- fieldNames (List[str]): 템플릿에 표시되는 병합 필드 이름의 목록입니다.
- fieldValues (List[str]): 템플릿에서 병합 필드를 대체할 값 목록입니다.
편지 병합을 수행하는 단계
- 편지 병합 템플릿 로드: 문서 클래스 개체를 인스턴스화하고 편지 병합 템플릿을 로드합니다.
- 데이터 소스 정의: 템플릿에 삽입할 특정 세부 정보가 포함된 사전을 준비합니다. 사전의 키는 템플릿에 정의된 병합 필드의 이름과 일치해야 합니다.
- 편지 병합 실행: Document.MailMerge.Execute() 메서드를 사용하여 템플릿의 병합 필드를 데이터 소스의 해당 값으로 바꿉니다.
- 문서 저장하기: 병합된 데이터가 포함된 최종 문서를 Document.SaveToFile() 메서드를 사용하여 저장합니다.
다음은 문서 병합 템플릿을 데이터로 채우기 위해 Document.MailMerge.Execute() 메서드를 사용하는 방법을 보여주는 예시입니다.
from spire.doc import *
from spire.doc.common import *
# Create an object of the Document class
doc = Document()
# Load the mail merge template
doc.LoadFromFile("MailMergeTemplate.docx")
# Define the data source as a dictionary
# In the dictionary, the keys represent the names of the merge fields used in the mail merge template, and the values represent the corresponding data that will be inserted into those merge fields.
data_source = {
"CompanyName": "Global Solutions",
"CompanyAddress": "456 Oak Avenue",
"FirstName": "Mary",
"LastName": "Johnson",
"EventLocation": "City Hall",
"EventDate": "2024-09-20",
"SenderName": "Jane Smith",
"SenderPosition": "Director of Events"
}
# Replace the merge fields in the mail merge template with the corresponding values
doc.MailMerge.Execute(list(data_source.keys()), list(data_source.values())
# Save the merged document
doc.SaveToFile("output/MergedDocument.docx", FileFormat.Docx2016)
# Close the document
doc.Close()
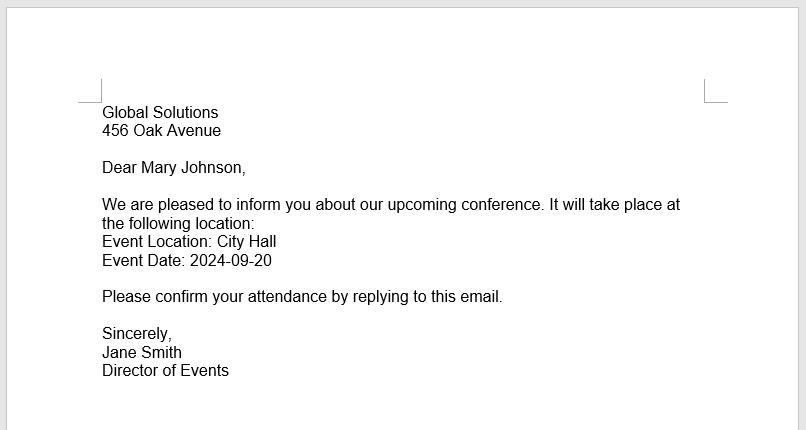
파이썬으로 Word에서 편지 병합을 사용하여 한 번에 여러 문서 만들기
이전 예제에서는 편지 병합 템플릿에서 병합된 단일 문서를 만들었습니다. 많은 수의 수신자를 처리할 때는 각 수신자에 대해 개별화된 별도의 문서를 생성해야 하는 경우가 많습니다. 이렇게 하면 받는 사람의 이름, 주소 및 기타 개인 정보와 같은 특정 세부 정보로 각 문서를 맞춤 설정할 수 있습니다.
- 편지 병합 템플릿 로드: 문서 클래스 개체를 인스턴스화하고 편지 병합 템플릿을 로드합니다.
- 데이터 소스 정의: 각 사전이 한 명의 수신자에 대한 데이터를 포함하는 사전 목록을 준비합니다. 사전의 키는 템플릿에 정의된 병합 필드의 이름과 일치해야 합니다.
- 데이터 소스 반복: 데이터 소스의 각 딕셔너리에 대해 반복합니다.
- 템플릿 복제: 편지 병합 템플릿의 사본을 만듭니다.
- 편지 병합 실행: 복제된 문서의 병합 필드를 사전의 해당 값으로 바꿉니다.
- 문서 저장하기: 개인화된 문서를 별도의 파일로 저장합니다.
다음은 Python으로 Word에서 편지 병합을 사용하여 한 번에 여러 문서를 만드는 방법을 보여주는 예제입니다.
from spire.doc import *
from spire.doc.common import *
# Create an object of the Document class
doc = Document()
# Load the mail merge template
doc.LoadFromFile("MailMergeTemplate.docx")
# Define the data source as a list of dictionaries
# Each dictionary contains the data for one recipient
data_source = [
{
"CompanyName": "Acme Corporation",
"CompanyAddress": "123 Elm Street",
"FirstName": "John",
"LastName": "Doe",
"EventLocation": "Conference Center",
"EventDate": "2024-09-15",
"SenderName": "Jane Smith",
"SenderPosition": "Director of Events"
},
{
"CompanyName": "Global Solutions",
"CompanyAddress": "456 Oak Avenue",
"FirstName": "Mary",
"LastName": "Johnson",
"EventLocation": "City Hall",
"EventDate": "2024-09-20",
"SenderName": "Jane Smith",
"SenderPosition": "Director of Events"
},
{
"CompanyName": "Tech Innovators",
"CompanyAddress": "789 Pine Road",
"FirstName": "James",
"LastName": "Williams",
"EventLocation": "Community Center",
"EventDate": "2024-09-25",
"SenderName": "Jane Smith",
"SenderPosition": "Director of Events"
}
]
# Loop through the data source
for entry in data_source:
# Create a copy of the template
clone_doc = doc.Clone()
# Replace the merge fields in the copied document with the corresponding values
doc.MailMerge.Execute(list(entry.keys()), list(entry.values()))
# Save the copied document to a separate file
clone_doc.SaveToFile(f"output/Members/Member-{entry['FirstName']}_{entry['LastName']}.docx")
doc.Close()
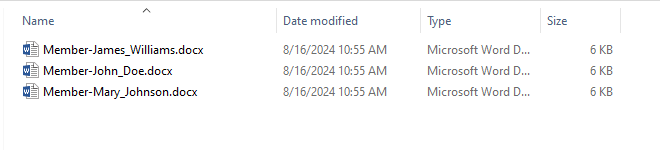
파이썬으로 Word에서 병합 필드 이름 검색하기
Spire.Doc을 사용하여 편지 병합 템플릿에서 병합 그룹 및 병합 필드의 이름을 검색할 수 있습니다.
- 편지 병합 템플릿 로드: 문서 클래스 개체를 인스턴스화하고 편지 병합 템플릿을 로드합니다.
- 그룹 이름 검색: 병합 그룹의 이름을 가져옵니다.
- 병합 필드 이름 검색: 특정 그룹 내의 병합 필드 이름을 가져오거나 템플릿 내의 모든 병합 필드 이름을 가져옵니다.
다음은 Python으로 편지 병합 템플릿에서 병합 그룹 및 병합 필드의 이름을 검색하는 방법을 보여주는 예제입니다.
from spire.doc import *
from spire.doc.common import *
# Create a Document object and load the mail merge template
doc = Document()
doc.LoadFromFile("MailMergeTemplate.docx")
# Get the collection of group names
group_names = doc.MailMerge.GetMergeGroupNames()
# Get the collection of merge field names within a specific group such as "Products"
merge_field_names_within_group = doc.MailMerge.GetMergeFieldNames("Products")
# Get the collection of all merge field names
merge_field_names = doc.MailMerge.GetMergeFieldNames()
# Prepare content for output
content = []
# Append group names if not empty
if group_names:
content.append("-------------- Group Names ----------------------")
content.extend(group_names)
# Append merge field names within a specific group if not empty
if merge_field_names_within_group:
content.append("-------- Merge Field Names Within Specific Group ---------")
content.extend(merge_field_names_within_group)
# Append all merge field names if not empty
if merge_field_names:
content.append("---------- All Merge Field Names -----------------")
content.extend(merge_field_names)
# Write the contents to a TXT file
output_file = "output/merge_fields.txt"
with open(output_file, mode="w", encoding="utf-8") as f:
f.write("\n".join(content))
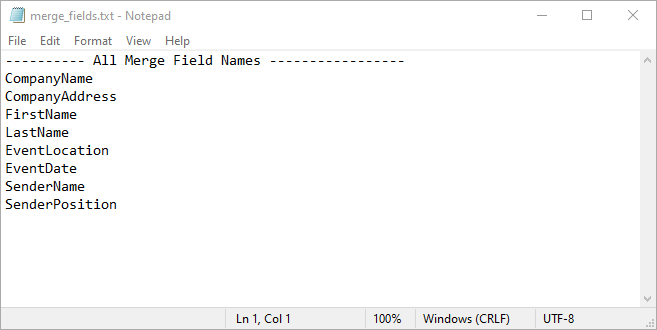
마치며
Excel과 Word를 이용하여 메일 병합을 할 수도 있지만 파이썬을 사용하여 Word에서 메일 병합 템플릿을 만들고 메일 병합을 할 수 있습니다.
'Python' 카테고리의 다른 글
파이썬을 사용하여 Excel 피벗 테이블 다루기 (1) | 2024.11.09 |
---|---|
파이썬에서 PDF에 디지털 서명을 추가하는 6가지 방법 (3) | 2024.11.03 |
파이썬을 사용하여 Excel에서 데이터 유효성 검사 사용하는 방법 (7) | 2024.10.03 |
파이썬에서 PDF를 Word로 변환하는 5가지 방법 (5) | 2024.10.01 |
파이썬을 사용하여 PDF에서 이미지와 이미지 정보 추출하기 (2) | 2024.09.29 |